Laravel Job not recognizing object property
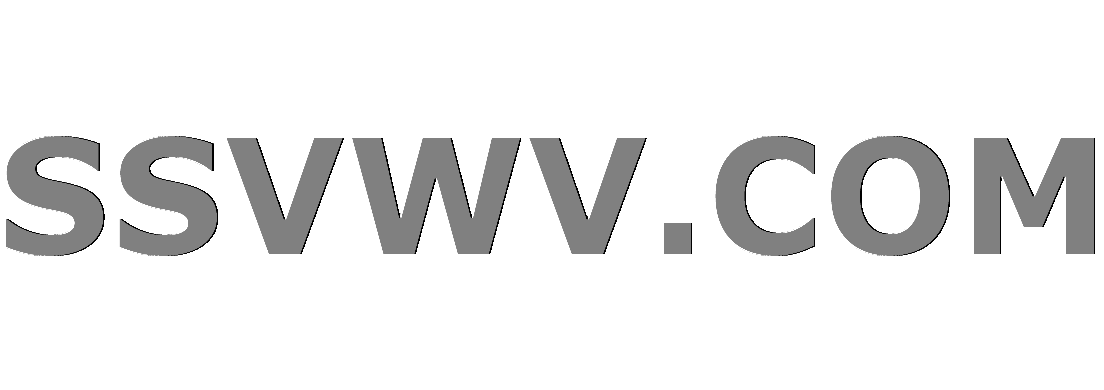
Multi tool use
Laravel Job not recognizing object property
I am passing a request to a job class to send an email for a contact form. The issue is that I cannot directly access the property.
I set the $request in the constructor as $this->request = $request;
$this->request = $request;
In the handle()
method, I do this:
handle()
Log::info( 'job-contact-request', [ 'request' => $this->request ] );
the log spits out:
[2018-06-30 14:07:34] local.INFO: request {"request":"[object] (AppEventsContactRequestValidated: {"request":{"client_name":"Daniel","client_email":"email@email.com","client_phone":"1234567891","client_text":"blargle carble darble zarble"},"socket":null})"}
Which shows that the client_email is set. But when I try to access $this->request->client_email
, I get:
$this->request->client_email
[2018-06-30 14:15:30] local.ERROR: Undefined property: AppEventsContactRequestValidated::$client_email {"exception":"[object] (ErrorException(code: 0): Undefined property: AppEventsContactRequestValidated::$client_email at /var/www/site/laravel/app/Jobs/SendContactRequestEmail.php:36)
[stacktrace]
@Marcus I figured out the issue in my answer below.
– Daniel Foust
Jul 1 at 13:36
1 Answer
1
Just messing with Laravel events/listeners/jobs, there were a few things I had to correct. Having logs across multiple classes was really throwing me off.
In the Event constructor, I had to use the all()
method when setting the request.
all()
$this->request = $request->all()
to properly access the data by property name.
$this->request = $request->all()
// Event Constructor
public function __construct( ContactRequest $request )
{
$this->request = $request->all();
// Log::info( 'Event-Constructor', [ 'contact_request' => $this->request ] );
}
The event passes the listener an event object with the data set in the event constructor as a property of an event object. The data has to be accessed via the event object.
// Listener
public function handle( ContactRequestValidated $event )
{
// Log::info( 'listener', [ 'contact-event' => $event->request ] );
dispatch( new SendContactRequestEmail( $event->request ));
}
The next part I'm not really sure why it is happening, but in the job, the request was changed from an object to an array.
So this is the job code:
// Job Constructor
public function __construct( $request )
{
$this->request = $request; // Actually an array, not an object
}
// Job handle
public function handle()
{
// Log::info( 'job-contact-request', [ 'request' => $this->request ] );
$email = new ConfirmContactRequest( $this->request );
Log::info( 'job-contact-request', [ 'email_html' => $email ] );
// Note request has to be accessed by array notation rather than arrow method
Mail::to( $this->request['client_email'] )->send( $email );
}
Is this a registered listener? Your handle method receives the event as its first parameter. I may be wrong but I see no reason to use the constructor. Following what I mentioned seems to be the "Laravel way"
– OneLiner
Jul 1 at 16:29
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Can you share the code for it?
– Marcus
Jul 1 at 13:30