Pygame - locking the scroll of a surface area to a limited amount
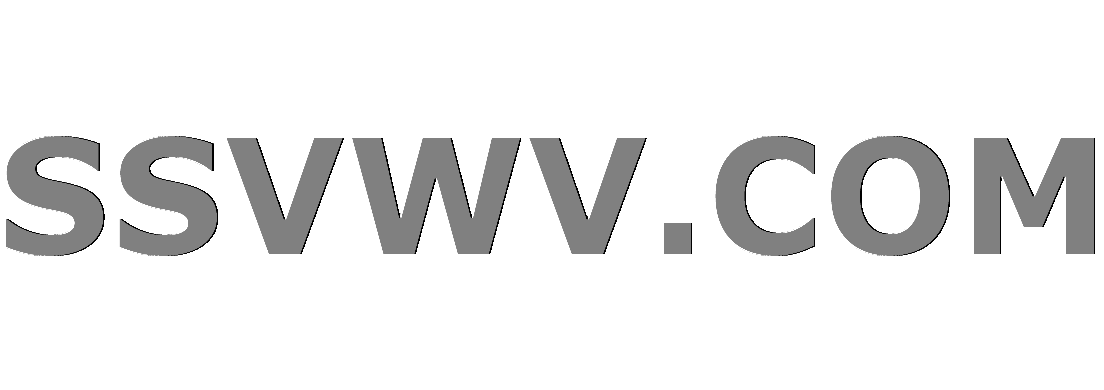
Multi tool use
Pygame - locking the scroll of a surface area to a limited amount
I'm playing with pygame and I'm making a memory game.
In my memory game I need to have a small Menu on the middle-bottom of the screen and the player list on top of the screen. The game manager will output the message in the upper left corner of the screen.
The playable board where the cards will be situated should be in the center of the screen with a small margin on the right and the left and about 200px from the top and the bottom.(e.g. if the screen width and height are 1200 x 800, the playable board should be from 2 to 1998 width and 200 to 600 height.
I've made a screenshot of the idea I have:
I've put up the idea of the scrollable board with the cards, although it's hardcoded for now, I mostly need the idea not the code since I just have a hard time figuring out how it should work.
The playable board (game board on the screenshot), should be moved through the keyboard(so up, right, down, left arrows). The thing I want to do is to allow more cards than 4 in each column and 12 in each row, in a way that when there are more cards than the 12 x 4, the gameboard could be moved, but without exiting the red square marked on the screenshot(so it's a scrollable surface). I want to limit the scrollable area to a width of 1200 and height of 400 px's, but in a way that the area is scrollable only inside the red area.
If that can't work or is planned otherwise, is there any way to register clicks differently? Let's say when I move the keys in a way that the Menu area is above the game board and the menu is clicked, I don't want the card under the Menu to be clicked as well. Is there any way to lock the content under the Menu?
Adding the code sample below:
from pygame import *
import sys
init()
screen_width = 1200
screen_height = 800
screen = display.set_mode((screen_width, screen_height))
board_width = 2000
board_height = 1000
playable_board = Surface((board_width, board_height))
playable_board = playable_board.convert()
screen.fill((255, 255, 255))
#draw.rect(playable_board, (125, 125, 125), (2, 200, board_width, board_height))
for x in range(0, 50):
draw.rect(playable_board, (100, 100, 100), (x * 100 + 5, 200, 90, 90))
draw.rect(playable_board, (100, 100, 100), (x * 100 + 5, 310, 90, 90))
draw.rect(playable_board, (100, 100, 100), (x * 100 + 5, 420, 90, 90))
draw.rect(playable_board, (100, 100, 100), (x * 100 + 5, 530, 90, 90))
# draw.rect(playable_board, (100, 100, 100), (x * 100 + 5, 640, 90, 90))
point_x = 0
point_y = 0
point_change = -30
animationTimer = time.Clock()
endProgram = False
while not endProgram:
for e in event.get():
pass
key_pressed = key.get_pressed()
if e.type == QUIT:
endProgram = True
if e.type == MOUSEBUTTONUP:
mouse_x, mouse_y = e.pos
display.set_caption(('Coordinates: ' + str(mouse_x + abs(point_x)) + 'x' + str(mouse_y + abs(point_y))))
draw.rect(playable_board, (50, 200, 50), (mouse_x + abs(point_x), mouse_y + abs(point_y), 10, 10))
if key_pressed[K_LEFT]:
point_x -= point_change
if key_pressed[K_RIGHT]:
point_x += point_change
if key_pressed[K_UP]:
point_y -= point_change
if key_pressed[K_DOWN]:
point_y += point_change
if point_x > 0:
point_x = 0
if point_x < -(board_width - screen_width):
point_x = -(board_width - screen_width)
if point_y > 0:
point_y = 0
if point_y < -(board_height - screen_height):
point_y = -(board_height - screen_height)
screen.blit(playable_board, (point_x, point_y, screen_width, screen_height))
draw.rect(screen, (100, 255, 100), (500, 650, 200, 150))
draw.rect(screen, (100, 255, 100), (100, 0, 1000, 50))
draw.rect(screen, (100, 255, 100), (0, 70, 250, 100))
animationTimer.tick(60)
display.update()
1 Answer
1
I'd create a subsurface of the playable_board
and then blit it at the desired coordinates. You need to define a rect (called area
here) with the desired size of the subsurface and scroll it by incrementing the x
and y
attributes. To keep the area
rect inside of the board_rect
(to prevent ValueError
s), you can call the pygame.Rect.clamp
or clamp_ip
methods.
playable_board
area
x
y
area
board_rect
ValueError
pygame.Rect.clamp
clamp_ip
import pygame as pg
pg.init()
screen = pg.display.set_mode((1200, 800))
board = pg.Surface((2000, 1000))
board.fill((20, 70, 110))
board_rect = board.get_rect()
# Append the rects to a list if you want to use
# them for collision detection.
rects =
for y in range(11):
for x in range(22):
rect = pg.draw.rect(board, (100, 100, 100), (x*95, y*95, 90, 90))
rects.append(rect)
# The blit position of the subsurface.
pos = (20, 200)
point_change = -10
# This rect is needed to create a subsurface with the size (1160, 400).
area = pg.Rect(0, 0, 1160, 400)
# Use the area rect to create a subsurface of the board.
board_subsurface = board.subsurface(area)
clock = pg.time.Clock()
endProgram = False
while not endProgram:
for e in pg.event.get():
if e.type == pg.QUIT:
endProgram = True
key_pressed = pg.key.get_pressed()
# Scroll by changing the x and y coordinates of the area rect.
if key_pressed[pg.K_LEFT]:
area.x += point_change
elif key_pressed[pg.K_RIGHT]:
area.x -= point_change
if key_pressed[pg.K_UP]:
area.y += point_change
elif key_pressed[pg.K_DOWN]:
area.y -= point_change
# Check if one of the movement keys was pressed.
if any(key_pressed[key] for key in (pg.K_LEFT, pg.K_RIGHT, pg.K_UP, pg.K_DOWN)):
# Clamp the area rect to the board_rect, otherwise calling
# `board.subsurface` could raise a ValueError.
area.clamp_ip(board_rect)
# Create a new subsurface with the size of the area rect.
board_subsurface = board.subsurface(area)
screen.fill((30, 30, 30))
screen.blit(board_subsurface, pos)
pg.draw.rect(screen, (100, 255, 100), (500, 650, 200, 150))
pg.draw.rect(screen, (100, 255, 100), (100, 0, 1000, 50))
pg.draw.rect(screen, (100, 255, 100), (0, 70, 250, 100))
clock.tick(60)
pg.display.update()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.