Audio Livestreaming with Python & Flask
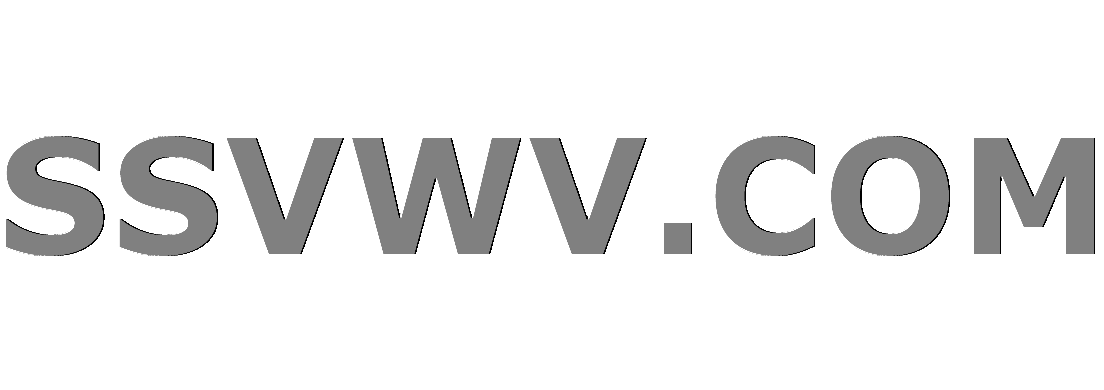
Multi tool use
Audio Livestreaming with Python & Flask
I'm currently strugling with my implementation of a simple live streaming web application using Python and Flask.
It seems that I'm not able to stream my live recorded audio from the servers microphone input to the webpage.
server.py
from flask import Flask, render_template, Response
import cv2
import framework
import pyaudio
import audio_processing as audioRec
FORMAT = pyaudio.paInt16
CHANNELS = 1
RATE = 44100
CHUNK = 1024
audio = pyaudio.PyAudio()
app = Flask(__name__)
@app.route('/')
def index():
"""Video streaming home page."""
return render_template('index.html')
# Stream routing
@app.route('/video_feed')
def video_feed():
"""Video streaming route. Put this in the src attribute of an img tag."""
return Response(generateVideo(),
mimetype='multipart/x-mixed-replace; boundary=frame')
@app.route("/audio_feed")
def audio_feed():
"""Audio streaming route. Put this in the src attribute of an audio tag."""
return Response(generateAudio(),
mimetype="audio/x-wav")
# Stream generating
def generateVideo():
"""Video streaming generator function."""
cap = cv2.VideoCapture(0)
while (cap.isOpened()):
ret, frame = cap.read()
output = framework.streamer(frame, 'final')
cv2.imwrite('signals/currFrame.jpg', output)
yield (b'--framern'
b'Content-Type: image/jpegrnrn' + open('signals/currFrame.jpg', 'rb').read() + b'rn')
def generateAudio():
"""Audio streaming generator function."""
currChunk = audioRec.record()
data_to_stream = genHeader(44100, 32, 1, 200000) + currChunk
yield data_to_stream
# with open("signals/audio.wav", "rb") as fwav:
# data = fwav.read(1024)
# while data:
# yield data
# data = fwav.read(1024)
def genHeader(sampleRate, bitsPerSample, channels, samples):
datasize = samples * channels * bitsPerSample // 8
o = bytes("RIFF",'ascii') # (4byte) Marks file as RIFF
o += (datasize + 36).to_bytes(4,'little') # (4byte) File size in bytes excluding this and RIFF marker
o += bytes("WAVE",'ascii') # (4byte) File type
o += bytes("fmt ",'ascii') # (4byte) Format Chunk Marker
o += (16).to_bytes(4,'little') # (4byte) Length of above format data
o += (1).to_bytes(2,'little') # (2byte) Format type (1 - PCM)
o += (channels).to_bytes(2,'little') # (2byte)
o += (sampleRate).to_bytes(4,'little') # (4byte)
o += (sampleRate * channels * bitsPerSample // 8).to_bytes(4,'little') # (4byte)
o += (channels * bitsPerSample // 8).to_bytes(2,'little') # (2byte)
o += (bitsPerSample).to_bytes(2,'little') # (2byte)
o += bytes("data",'ascii') # (4byte) Data Chunk Marker
o += (datasize).to_bytes(4,'little') # (4byte) Data size in bytes
return o
if __name__ == '__main__':
app.run(host='0.0.0.0', debug=True, threaded=True)
audio_processing.py
import pyaudio
FORMAT = pyaudio.paInt16
CHANNELS = 1
RATE = 44100
CHUNK = 1024
audio = pyaudio.PyAudio()
def record():
# start Recording
stream = audio.open(format=FORMAT, channels=CHANNELS,
rate=RATE, input=True,
frames_per_buffer=CHUNK)
# print "recording..."
data = stream.read(CHUNK)
return data
I'm trying to get the current Chunk of the microphone using the audio_processing.py and using yield to respond the current samples to the user. The video stream works quite good.
Anyone knows, what I'm doing wrong here?
Kind regards,
Felix
record()
Yes, your are right, I changed the code a bit. I recently added the pyAudio recording procedure to the server.py into generateAudio(). The function should work properly right now. The only problem is, that the byte() function is not supported in Python2.7 which I am currently using. Do you have any idea, how to write a similar function in 2.7? Couldn't find a premade solution :/
– F. Geißler
Jun 28 at 14:26
1 Answer
1
The solution is here Audio streaming with flask
I am still working on it. (python version should be 3+)
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Just a quick note, you shouldn't be continuously opening the PyAudio stream; i.e. set it up in an init function call or something similar, before the streaming starts. Then access the stream object to read from it when you need with your
record()
method. Or at least close it if you want to keep opening it.– WoodyDev
Jun 28 at 9:50