Eigen and huge dense 2D arrays
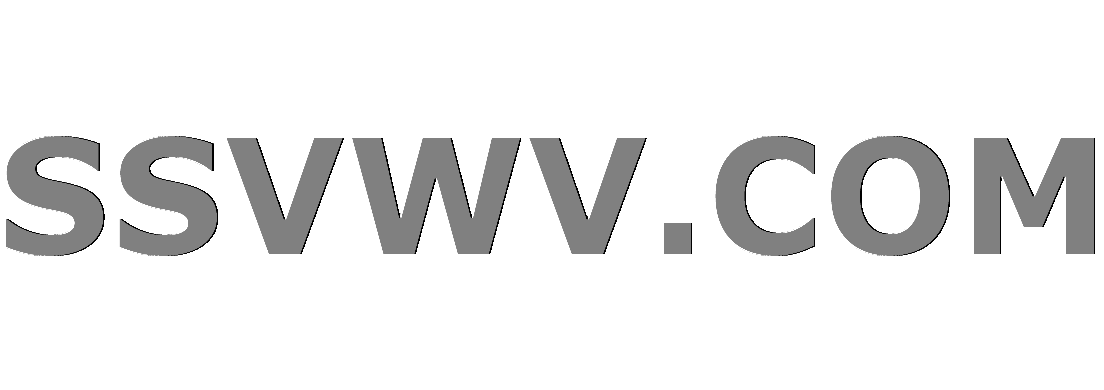
Multi tool use
Eigen and huge dense 2D arrays
I am using 2D Eigen::Array
s for a project, and I like to keep using them in the case of huge 2D arrays.
Eigen::Array
For avoiding memory issues, I thought to use memory mapped files to manage (read/modify/write) these arrays, but I cannot find working examples.
The closest example that I have found is this based on boost::interprocess
, but it uses shared-memory (while I'd prefer to have persistent storage).
boost::interprocess
The lack of examples makes me worry if there is a better, main-stream alternative solution to my problem. Is this the case? A minimal example would be very handy.
EDIT:
This is a minimal example explaining my use case in the comments:
#include <Eigen/Dense>
int main()
{
// Order of magnitude of the required arrays
Eigen::Index rows = 50000;
Eigen::Index cols = 40000;
{
// Array creation (this is where the memory mapped file should be created)
Eigen::ArrayXXf arr1 = Eigen::ArrayXXf::Zero( rows, cols );
// Some operations on the array
for(Eigen::Index i = 0; i < rows; ++i)
{
for(Eigen::Index j = 0; j < cols; ++j)
{
arr1( i, j ) = float(i * j);
}
}
// The array goes out of scope, but the data are persistently stored in the file
}
{
// This should actually use the data stored in the file
Eigen::ArrayXXf arr2 = Eigen::ArrayXXf::Zero( rows, cols );
// Manipulation of the array data
for(Eigen::Index i = 0; i < rows; ++i)
{
for(Eigen::Index j = 0; j < cols; ++j)
{
arr2( i, j ) += 1.0f;
}
}
// The array goes out of scope, but the data are persistently stored in the file
}
}
This question has not received enough attention.
A simple working example would be enough.
@Darklighter, could you develop a bit more your comment?
– gmas80
Jun 30 at 21:36
Instead of trying to use a file as a chunk of memory you can simply expand your virtual memory via the page/swap file. This allows you to use matrices that are bigger than your physical memory. It probably only works reasonably well on an SSD though.
– Darklighter
Jul 1 at 1:14
As you said yourself, a Minimal, Complete, and Verifiable example of what you actually intend to do would be very handy. Or at least some pseudo-code. Do you have existing arrays stored, which you want to traverse linearly/access randomly? Or do you generate huge arrays at runtime which are just too large to fit in your RAM? What orders of magnitude are you working with?
– chtz
Jul 1 at 6:42
You should be able to come up with something using boost::interprocess to map a file as a memory buffer, and then (Eigen::Map)[eigen.tuxfamily.org/dox/group__TutorialMapClass.html] to view it and manipulate it as an
ArrayXXf
.– ggael
2 days ago
ArrayXXf
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
you can create huge swap files and have the OS swap pages as necessary
– Darklighter
Jun 30 at 20:06