C++ thread error: no type named ‘type’ MINGW
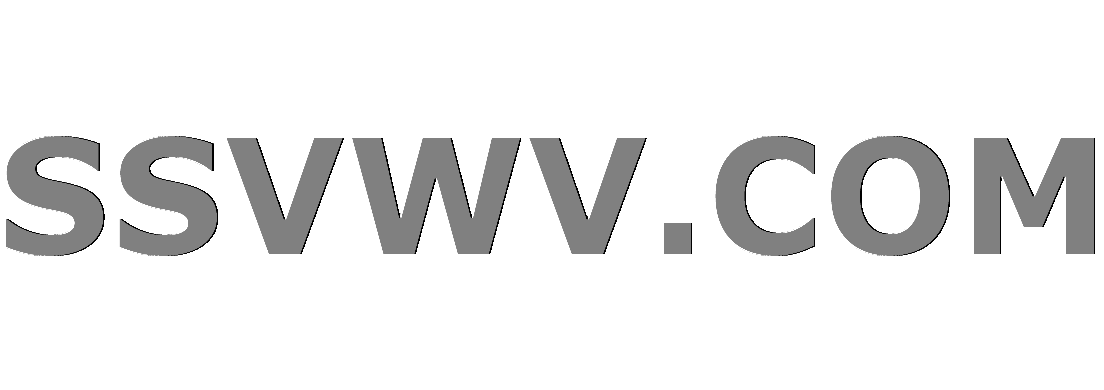
Multi tool use
C++ thread error: no type named ‘type’ MINGW
I'm currently doing a project for my operational systems class and I have to make a program to find prime numbers to run in threads.
So I did this:
#include <iostream>
#include <cmath>
#include <thread>
#define THREADNUMBER 100
using namespace std;
int CONTADOR = 0;
int CONTADORTHREADEXECUTADA = 0;
int primeRange(int v1, int v2) {
int a, limit;
bool isprime;
for (int i = v1; i <= v2; i++) {
if (i % 2 != 0 && i % 3 != 0) {
isprime = true;
limit = i / 2;
if(i == 1) isprime =false;
limit = (int)sqrt(i); //General case
for(a=2; a <= limit; a++){
if(i % i == 0 && i != 2){
isprime = false;
break;
}
}
if (isprime) {
CONTADOR++;
}
}
}
CONTADORTHREADEXECUTADA++;
return 1;
}
int main(int argc, char *argv[ ] ) {
int number1 = atoi(argv[1]);
int number2 = atoi(argv[2]);
int dif = number2-number1;
thread** vec = new thread*[THREADNUMBER];
cout<< "criando threads" <<endl;
int contadorthread = 0;
if (dif <= THREADNUMBER) {
for(int i = number1; i <= number2; i++) {
thread* t = new thread(primeRange(i,i));
vec[contadorthread] = t;
contadorthread++;
}
} else {
int c = dif / THREADNUMBER;
for(int i = number1; i <= number2; i+=(c+1)) {
if (contadorthread==THREADNUMBER-1) {
thread* t = new thread(primeRange(i,number2));
vec[contadorthread] = t;
contadorthread++;
break;
}
thread* t = new thread(primeRange(i,i+c));
vec[contadorthread] = t;
contadorthread++;
}
cout<<contadorthread << " threads criadas"<<endl;
cout<<"inicializando threads" <<endl;
while (CONTADORTHREADEXECUTADA < contadorthread) {
cout<<contadorthread - CONTADORTHREADEXECUTADA << endl;
}
cout<< CONTADOR << "primos encontrados" <<endl;
}
}
But everytime I try to compile on 64 windows GCC I get this error message saying that there is not type named 'type'
I compile with this
g++ -Wall -g -std=c++11 -pthread codigo.cpp -o exe -Wall
What can I do?
I already downloaded the version of mingw they say is thread safe.
BTW you are leaking thread objects. Why are you using all those
new
and naked pointers? Just put plain std::thread
objects straight into an std::vector
.– Matteo Italia
2 days ago
new
std::thread
std::vector
Please show exactly what build of mingw-w64 you are using (I presume you are actually using mingw-w64 and not mingw). Some of them do not actually have std thread support out-of-the-box
– M.M
2 days ago
@MatteoItalia IMO it would be preferable to use a higher level abstraction over
std::thread
, e.g. packaged_task
or async
– M.M
2 days ago
std::thread
packaged_task
async
This message usually means you are trying to instantiate a template incorrectly. (It's not a good error message but that's how templates work right now). The template in your case is the std::thread constructor.
– n.m.
2 days ago
1 Answer
1
The statement
thread* t = new thread(primeRange(i,i));
call function primeRange and pass result(int) to thread constructor which is obviously not the way to call. Instead use:
thread* t = new thread(primeRange, i, i);
It will pass function pointer as well as arguments to thread constructor.
Thanks! I actually got the answer from a friend, but was very busy with the job. But it hoped, thank you again.
– Koda
2 days ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Please, add exact error message into the question post. If the error message contain the line number, show that line in your code.
– Tsyvarev
Jun 30 at 22:32