Why do i have to dereference a reference (not a pointer) to access its data (context: used as parameter in STL-algorithm)?
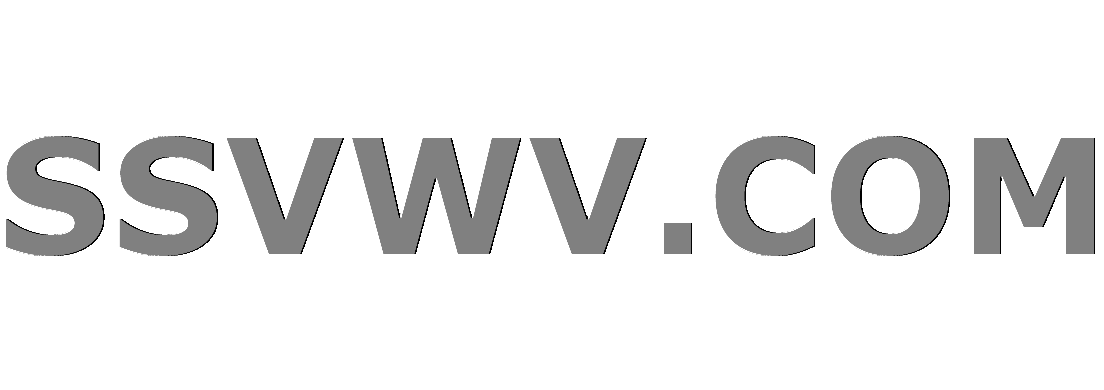
Multi tool use
Why do i have to dereference a reference (not a pointer) to access its data (context: used as parameter in STL-algorithm)?
I'm writing a programm that's supposed to define classes that represent a sparse matrix (matrix with few non-zero values) and then perform a few operations on a vector of these sparse matrixes. The part where i had to define the classes mostly works (1) and there also already is a working vector of the matrices ("matrixvec") itself. However now i just want to add all the matrices together using the accumulate-algorithm from the STL.
This works somewhat, but not really in the way i expected. So basically i give accumulate as an initial value an external matrix called "sammelmatrix" and then accumulate adds every matrix to "sammelmatrix" (using a self-defined +operator (that works in other parts of the programm too). Using this method (instead of e.g. "sammelmatrix = accumulate(...);") i think you would need to pass sammelmatrix by-reference to the matrix so that sammelmatrix itselfs values are changed, not just that of the copy. However why do i need to dereference summe inside the lambda-expression, i thought a reference is just another name for the variable, so there is really nothing to dereference here (no space in memory to go to from the value as the value already is actual data)? Thanks in advance for any help.
SparseMatrix sammelmatrix;
accumulate(matrixvec.begin(), matrixvec.end(), &sammelmatrix, (auto summe, auto aktelement) {
*summe = *summe + aktelement; //summe = summe + aktelement doesn't work
return summe;
});
(1)There is a class "SparseMatrix" that offers some operations for the individual matrix and has a vector that stores the non-zero matrix-values. The vectors data elements are of the type "MatrixElement" that just define a matrix-element basically.
EDIT: See comments for answers/explainations.
accumulate
sammelmatrix
summe
SparseMatrix*
sammelmatrix
Why does it see "&sammelmatrix" in the parameter list as a pointer? (I don't see that in the general form of the accumulate-function (" template <class InputIterator, class T, class BinaryOperation> T accumulate (InputIterator first, InputIterator last, T init, BinaryOperation binary_op); ") or in the specific desription of init (init = Initial value for the accumulator) (source: cplusplus.com/reference/numeric/accumulate)).
– doe
Jun 30 at 19:26
Just realised that "&" is also the "address-of"-operator. (So currently there is an address in the parameter list where &sammelmatrix stands.) But how do i pass this variable by-reference then?
– doe
Jun 30 at 19:34
std::ref(sammelmatrix)
. Though at this point, you aren't really using accumulate
the way it's supposed to be used; you could just as well use for_each
and avoid the confusion.– Igor Tandetnik
Jun 30 at 20:05
std::ref(sammelmatrix)
accumulate
for_each
Thanks ,,,,,,,,,,,,,,,,,,,,
– doe
Jun 30 at 23:12
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
"i give accumulate as an initial value an external matrix called "sammelmatrix"" No. You give
accumulate
as an initial value a pointer tosammelmatrix
. So the first parameter of your lambda,summe
, is also aSparseMatrix*
- a pointer. The algorithm just passes it along. You are not passingsammelmatrix
by reference.– Igor Tandetnik
Jun 30 at 18:57