How to declare and add items to an array in Python?
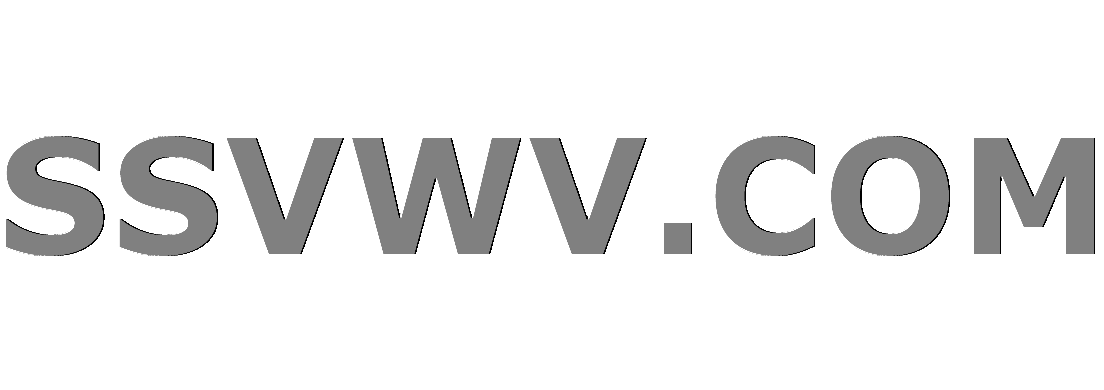
Multi tool use
How to declare and add items to an array in Python?
I'm trying to add items to an array in python.
I run
array = {}
Then, I try to add something to this array by doing:
array.append(valueToBeInserted)
There doesn't seem to be a .append
method for this. How do I add items to an array?
.append
4 Answers
4
{}
represents an empty dictionary, not an array/list. For lists or arrays, you need .
{}
To initialize an empty list do this:
my_list =
or
my_list = list()
To add elements to the list, use append
append
my_list.append(12)
To extend
the list to include the elements from another list use extend
extend
extend
my_list.extend([1,2,3,4])
my_list
--> [12,1,2,3,4]
To remove an element from a list use remove
remove
my_list.remove(2)
Dictionaries represent a collection of key/value pairs also known as an associative array or a map.
To initialize an empty dictionary use {}
or dict()
{}
dict()
Dictionaries have keys and values
my_dict = {'key':'value', 'another_key' : 0}
To extend a dictionary with the contents of another dictionary you may use the update
method
update
my_dict.update({'third_key' : 1})
To remove a value from a dictionary
del my_dict['key']
No, if you do:
array = {}
IN your example you are using array
as a dictionary, not an array. If you need an array, in Python you use lists:
array
array =
Then, to add items you do:
array.append('a')
Nitpicking: You don't declare anything. You make a name in the current scope refer to a dictionary/list object.
– user395760
May 7 '12 at 18:46
@delnan: thanks! changed the wording a little bit.
– Pablo Santa Cruz
May 7 '12 at 18:48
Arrays (called list
in python) use the notation.
{}
is for dict
(also called hash tables, associated arrays, etc in other languages) so you won't have 'append' for a dict.
list
{}
dict
If you actually want an array (list), use:
array =
array.append(valueToBeInserted)
In some languages like JAVA you define an array using curly braces as following but in python it has a different meaning:
Java:
int myIntArray = {1,2,3};
String myStringArray = {"a","b","c"};
However, in Python, curly braces are used to define dictionaries, which needs a key:value
assignment as {'a':1, 'b':2}
key:value
{'a':1, 'b':2}
To actually define an array (which is actually called list in python) you can do:
Python:
mylist = [1,2,3]
or other examples like:
mylist = list()
mylist.append(1)
mylist.append(2)
mylist.append(3)
print(mylist)
>>> [1,2,3]
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Oh ok, I understood it as a list would need to have a key and a value, but I guess not
– AkshaiShah
May 7 '12 at 18:44