while/try/except decimal TypeError not working
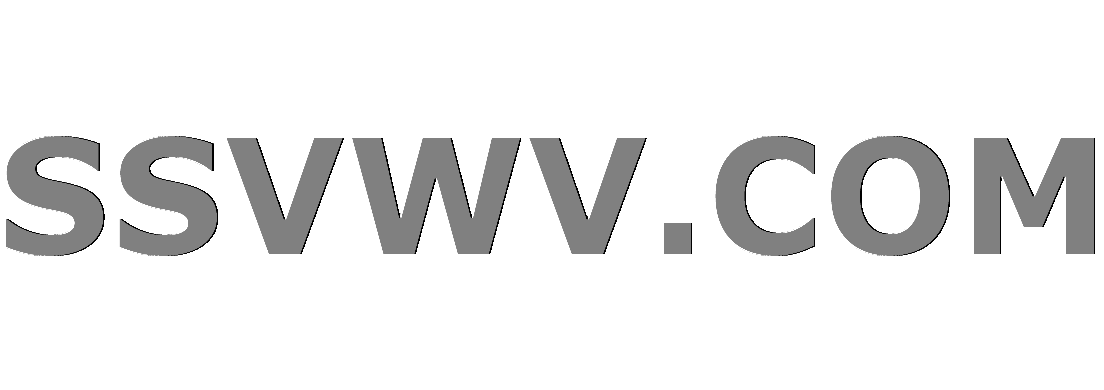
Multi tool use
while/try/except decimal TypeError not working
I thought I could finally get some use out of the while/try/except loop, but I am encountering a problem. My task is to have the computer request numbers from a user until '0' is entered. After this, the numbers entered will be added. My problem is that the Except part isn't catching non-numeric entries. If a non-numeric entry is entered, I get a regular error message that stops the program. Here is what happens when I enter 3 numbers and the 4th entry is 'm':
on win32
Type "copyright", "credits" or "license()" for more information.
>>>
RESTART: C:/Users/Username/Documents/CISPROG1/Homework 5/Homework5.1.SumGenerator.py
Enter 1st element (or type '0' to finish).
1
Enter 2nd element (or type '0' to finish).
2
Enter 3rd element (or type '0' to finish).
3
Enter 4th element (or type '0' to finish).
m
Traceback (most recent call last):
File "C:/Users/Username/Documents/CISPROG1/Homework 5/Homework5.1.SumGenerator.py", line 24, in <module>
+ "%s element (or type '0' to finish). n" % suffix()))
decimal.InvalidOperation: [<class 'decimal.ConversionSyntax'>]
>>>
I thought this would be a "TypeError" but that isn't catching it. In fact, not even "Exception" error type catches it. How do I catch non-numeric input? I think the problem has something to do with how Python handles non-numeric input for a Decimal type.
Here is my program:
from decimal import *
#start:
lInputSequence =
i = 1
def suffix():
if i%10 == 1 and i != 11:
return "st"
elif i%10 == 2 and i != 12:
return "nd"
elif i%10 == 3 and i != 13:
return "rd"
else:
return "th"
decInputElement = 1
while True:
decInputElement = Decimal(input("Enter " + str(i)
+ "%s element (or type '0' to finish). n" % suffix()))
try:
if decInputElement == 0:
break
else:
lInputSequence.append(decInputElement)
i = i+1
except TypeError:
print("Please enter a number.")
print(lInputSequence)
print("The sum of these %s elements is %s" % (len(lInputSequence),sum(lInputSequence)))
#end
EDIT 2: That works when I change the 'error type' to Exception. The type of error must not be TypeError. Thank you
– user391838
Jul 1 at 9:51
1 Answer
1
So, it looks like the problem is two-fold:
Solution:
This doesn't get to the root of what kind of error is coming up but it catches any input errors.
from decimal import *
#start:
lInputSequence =
i = 1
def suffix():
if i%10 == 1 and i != 11:
return "st"
elif i%10 == 2 and i != 12:
return "nd"
elif i%10 == 3 and i != 13:
return "rd"
else:
return "th"
decInputElement = 1
while True:
try:
decInputElement = Decimal(input("Enter " + str(i)
+ "%s element (or type '0' to finish). n" % suffix()))
if decInputElement == 0:
break
else:
lInputSequence.append(decInputElement)
i = i+1
except Exception:
print("Please enter a number.")
print(lInputSequence)
print("The sum of these %s elements is %s" % (len(lInputSequence),sum(lInputSequence)))
#end
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Your error is happening before the try statement, where you call Decimal on the input. Either move that call inside the try, it move the try.
– Daniel Roseman
Jul 1 at 9:47