componentDidMount not being called after goBack navigation call
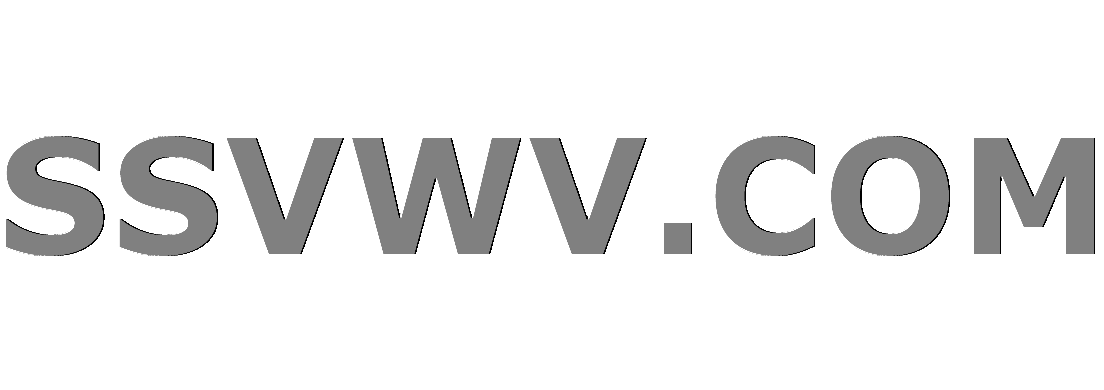
Multi tool use
componentDidMount not being called after goBack navigation call
I've set up a StackNavigator which will fire a redux action to fetch data on componentDidMount
, after navigating to another screen and going back to the previous screen, componentDidMount
is no longer firing and I'm presented with a white screen. I've tried to reset the StackNavigator using StackActions.reset
but that just leads to my other screens not mounting as well and in turn presenting an empty screen. Is there a way to force componentDidMount
after this.props.navigation.goBack()
?
componentDidMount
componentDidMount
StackActions.reset
componentDidMount
this.props.navigation.goBack()
Go Back Function
_goBack = () => {
this.props.navigation.goBack();
};
Navigation function to new screen
_showQuest = (questId, questTitle ) => {
this.props.navigation.navigate("Quest", {
questId,
questTitle,
});
};
componentDidMount
After navigating to a new screen,
componentDidMount
will be called the first time and after going back to the previous screen, it is no longer called. How do you forcefully unmount the screen after navigating to a new screen?– Ash Rhazaly
Jul 1 at 10:19
componentDidMount
1 Answer
1
The components are not removed when the navigation changes, so componentDidMount
will only be called the first time it is rendered.
componentDidMount
You could use this alternative approach instead:
class MyComponent extends React.Component {
state = {
isFocused: false
};
componentDidMount() {
this.subs = [
this.props.navigation.addListener("didFocus", () => this.setState({ isFocused: true })),
this.props.navigation.addListener("willBlur", () => this.setState({ isFocused: false }))
];
}
componentWillUnmount() {
this.subs.forEach(sub => sub.remove());
}
render() {
// ...
}
}
Sorry I recently migrated over from react-native router flux and i'm confused or rather not sure how to implement this approach. would isFocused be the boolean flag to trigger the redux action to fetch the data or navigate to a new screen?
– Ash Rhazaly
Jul 1 at 11:39
@AshRhazaly No worries. It's just an example, you don't have to use
isFocused
if you don't want to. The point is just that the didFocus
and willBlur
events can be seen as the componentDidMount
and componentWillUnmount
hooks in this case, since the component will never really be mounted/unmounted after the initial mount.– Tholle
Jul 1 at 12:09
isFocused
didFocus
willBlur
componentDidMount
componentWillUnmount
Do you have a working snack where I can see this implementation? I'm not fully sure how to go about this approach
– Ash Rhazaly
Jul 1 at 12:26
@AshRhazaly Sorry, I have no real experience with it myself. I only know what's written about it in the documentation.
– Tholle
Jul 1 at 12:30
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
How are you rendering your components? It might be that your other component is not actually unmounted when the navigation changes, and therefor
componentDidMount
will not be called again when you go back.– Tholle
Jul 1 at 9:53