Moq class with constructors ILogger and options netcore 2.1 vs2017 getting error
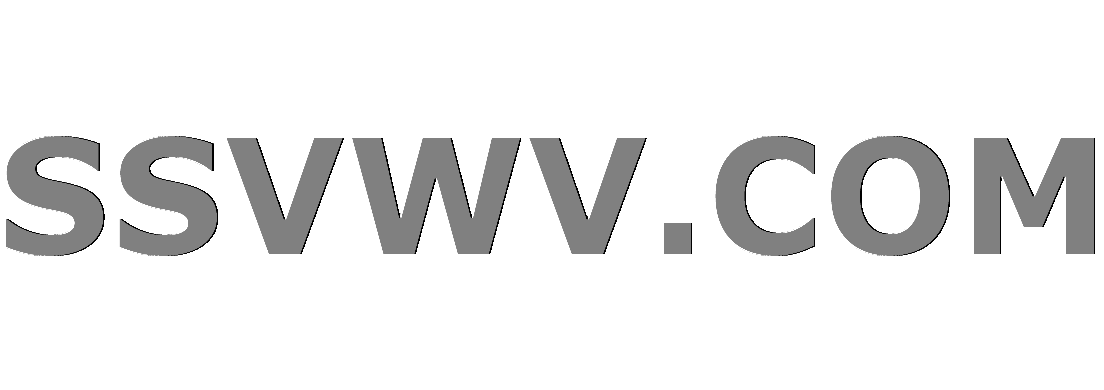
Multi tool use
Moq class with constructors ILogger and options netcore 2.1 vs2017 getting error
I need to mock a class that has parameters in the constructor by I cannot figure out how you do it using moq. It crashes
Constructor arguments cannot be passed for interface mocks.
See my attempt below:
[Fact]
public async Task MyTest()
{
var mySettings= GetMySettings();
var mySettingsOptions = Options.Create(mySettings);
var mockLogger = Mock.Of<ILogger<MyClass>>();
var mock=new Mock<IMyClass>(mySettings,mockLogger);
mock.Setup(x=>x.DoSomething(It.IsAny<string>().Returns("todo");
}
public class MyClass : IMyClass
{
private readonly ILogger<MyClass> logger;
private readonly MySettings mySettings;
public MyClass(IOptions<MySettings> settings,ILogger<MyClass>logger)
{
this.logger = logger;
this.mySettings = settings.Value;
}
public string DoSomething(string myarg)
{
//omitted
}
}
How do you do it? many thanks
EDITED
In order to mock repository and test the behaviour i also need to mock the other classes that have constructors in it. Hope makes sense
public class MyService:IMyService
{
private MyClass myclass;
private OtherClass otherClass;
private Repository repository;
public MyService(IRepository repository,IMyClass myclass,IMyOtherClass otherClass)
{
this.myclass=myClass;
this.otherClass=otherClass;
this.repository=repository;
}
public void DoStuff()
{
bool valid1=myclass.Validate(); //mock myclass
var valid2=otherClass.Validate(); //mock otherClass
if(Valid1 && valid2)
{
repository.GetSomething();//this is really what I am mocking
}
//etc..
}
}
@AlexRiabov i have edited my question
– developer9969
Jul 1 at 10:05
so you shouldn't care about constuctors for objects you're mocking
– Alex Riabov
Jul 1 at 10:13
2 Answers
2
It doesn't matter if your class constructor has parameters or not, because you're working with its mock object.
var mock = new Mock<IMyClass>();
mock.Setup(x=>x.DoSomething(It.IsAny<string>()).Returns("todo");
Then you can use this mock to your repository constructor:
var myService = new MyService(repositoryMock.Object, mock.Object, otherClassMock.Object);
you are right I was overcomplicating things for no reasons.Thank you
– developer9969
Jul 1 at 10:22
You are getting this error because you are trying to create a mock of an interface (IMyClass
in this case) with constructor values. It seems like you are trying to test the method in the class MyClass
, therefore you should be creating a moq of this class.
To clarify change var mock=new Mock<IMyClass>(mySettings,mockLogger);
to var mock=new Mock<MyClass>(mySettings,mockLogger);
IMyClass
MyClass
var mock=new Mock<IMyClass>(mySettings,mockLogger);
var mock=new Mock<MyClass>(mySettings,mockLogger);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What is actually your goal? Do you want to test DoSomething method? Or want to mock IMyClass to pass it to another class?
– Alex Riabov
Jul 1 at 9:54