Qt C++ “new” operation may cause unexpected errors?
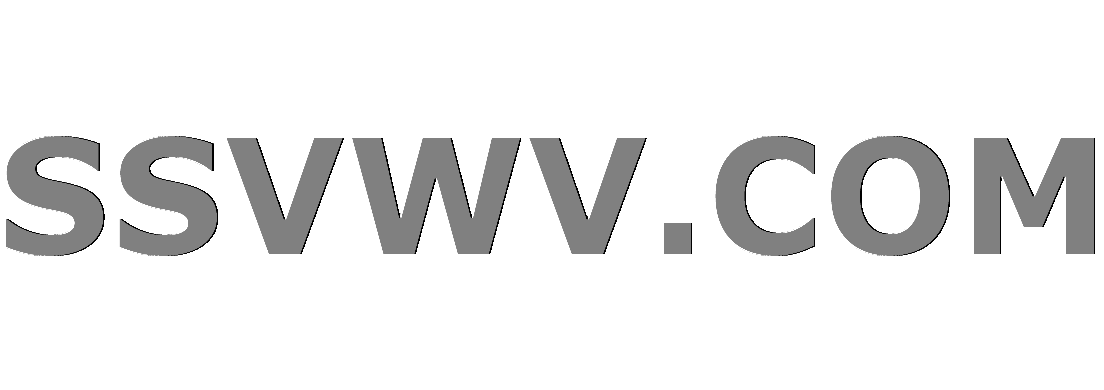
Multi tool use
Qt C++ “new” operation may cause unexpected errors?
There are two UIs in my project, namely, Login and MainWindow.
Now I use signal-slot method to transfer some data of Login to some MainWindow class member variables,and here is some key code:
Login::Login(QWidget *parent)
: QDialog(parent)
{
setupUi(this);
MainWindow *w = new MainWindow;
connect(this,SIGNAL(sendData(QList<QString>)),w,SLOT(receiveData(QList<QString>)));
}
//get data from Login
void MainWindow::receiveData(QList<QString> userList){
userName = userList.at(0);
password = userList.at(1);
QSqlQuery query;
bool b = query.exec(QString("SELECT * FROM user WHERE user_id = '%1' AND password = '%2'").arg(userName).arg(password));
if(b){
query.first();
userType = query.value(1).toString();
qDebug()<<"user_type:"<<userType; //always has value in userType
qDebug()<<"user_id:"<<userName; //always has value in userName
}
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
public:
QString userName; //member variables declared here.
QString password;
QString userType;
I tried to connect signal and slot in main function instead of Login constructor:
int main(int argc, char *argv) {
QApplication a(argc, argv);
...
MainWindow w;
Login l;
connect(&l,SIGNAL(sendData(QList<QString>)),&w,SLOT(receiveData(QList<QString>)));
if(l.exec() == QDialog::Accepted){
w.show();
}
return a.exec();
}
but with the following bug information:
D:QtProjectTMSmain.cpp:416: error: invalid conversion from 'Login*' to 'SOCKET {aka unsigned int}' [-fpermissive]
connect(&l,SIGNAL(sendData(QList<QString>)),&w,SLOT(receiveData(QList<QString>)));
D:QtProjectTMSmain.cpp:416: error: cannot convert 'const char*' to 'const sockaddr*' for argument '2' to 'int connect(SOCKET, const sockaddr*, int)'
Now, What really confuses me is that why the value of userName or userType is empty(which has been populated with data in function receiveData()) when I use them in other MainWindow functions.
I have tried searching on net for a long time but without any result working.
If you can give me any idea, I will appreciate it a lot.
Thanks in advance.
Login()
MainWindow
@AlexF I also have the similar idea about the question, but I don't know how to improve the code. Would you like give me some suggestions?
– chaotetung
Jun 24 at 14:14
@besc I guess so, but I'm a beginner and I don't know how to improve the code. if you can give me any suggestion,i will appreciate it a lot.
– chaotetung
Jun 24 at 14:20
2 Answers
2
What you tried in your main()
function is the right way to go. What you need to change:
main()
connect()
in main()
must be QObject::connect()
– it’s a static member function of the QObject
class. If you call it from inside the implementation of a class that derives from QObject
you don’t need to qualify the call because the compiler picks the correct function by default. Not so in main()
. From your compiler errors you can deduce that the compiler picked a completely unrelated connect()
function.
connect()
main()
QObject::connect()
QObject
QObject
main()
connect()
The constructor of Login
instantiates an additional MainWindow
object and connects to its slot. That’s not what you want. You want to use the existing main window object – w
. Delete the new and connect lines from Login::Login()
. They are redundant.
Login
MainWindow
w
Login::Login()
In main()
move return a.exec()
into the if
. Otherwise when the login dialog is not accepted your program does not show the main window, but it never terminates either. QApplication::exec()
starts the main GUI event loop. In a very tiny nutshell: That is what keeps your main window alive until the user decides to close the program. If you start the loop without showing the main window then there is no way to end the program except for killing the process. You want your main()
to look like this:
main()
return a.exec()
if
QApplication::exec()
main()
int main(int argc, char* argv)
{
QApplication app(argc, argv);
MainWindow mainWin;
Login loginDialog;
// ... connect and any other setup stuff
if (loginDialog.exec() == QDialog::Accepted) {
mainWin.show();
return app.exec();
}
return 1;
}
P.S. Avoid one-letter variable names. Without useful names you programs become unreadable extremely quickly.
your answer is very helpful and complete. I have learned a lot. Thanks.
– chaotetung
Jun 24 at 15:23
The error message indicates the source of the problem:
error: cannot convert 'const char*' to 'const sockaddr*' for argument '2' to 'int connect(SOCKET, const sockaddr*, int)'
You are calling a completely different connect()
function and not QObject::connect()
(notice the use of QObject
in front of the connect()
- it's very important since it's a static method). The sockaddr
indicates that you are trying to use the
connect()
QObject::connect()
QObject
connect()
sockaddr
int connect(int socket, const struct sockaddr *address, size_t address_len);
from #include <sys/socket.h>
, which is clearly incorrect. Perhaps you have added a header that replaces the QObject::connect()
when you do an autocomplete. I would suggest that you carefully check what your editor gives you as a suggestion and also check for the socket.h
header somewhere.
#include <sys/socket.h>
QObject::connect()
socket.h
I was able to reproduce an incorrect autocomplete by just adding the #include <sys/socket.h>
to a small Qt application and attempting to do an autocomplete inside Qt Creator inside the same source file:
#include <sys/socket.h>
Last but not least you really need to take care of the rest of the code. In addition I would also suggest using the new signal-slot syntax when creating a connection. It allows proper typechecking (the old SIGNAL()
and SLOT()
do not offer that since you just pass a string as argument).
SIGNAL()
SLOT()
What is the new signal-slot syntax? Thanks.
– chaotetung
Jun 24 at 15:07
your answer is helpful. thank you!
– chaotetung
Jun 24 at 15:24
@chaotetung Here is the new syntax.
– rbaleksandar
Jun 24 at 15:37
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Can you please post a MCVE. Your snippet is not enough to see clearly what’s going on. All I can say is that the
Login()
constructur reeks tremendously. Did you really want to create a newMainWindow
object in that constructor and store a local pointer to it? That pointer goes out of scope at the end of the ctor. That’s a memory leak right there. I suspect that line is part of the overall problem.– besc
Jun 24 at 14:03