unity c# - how to get values of list
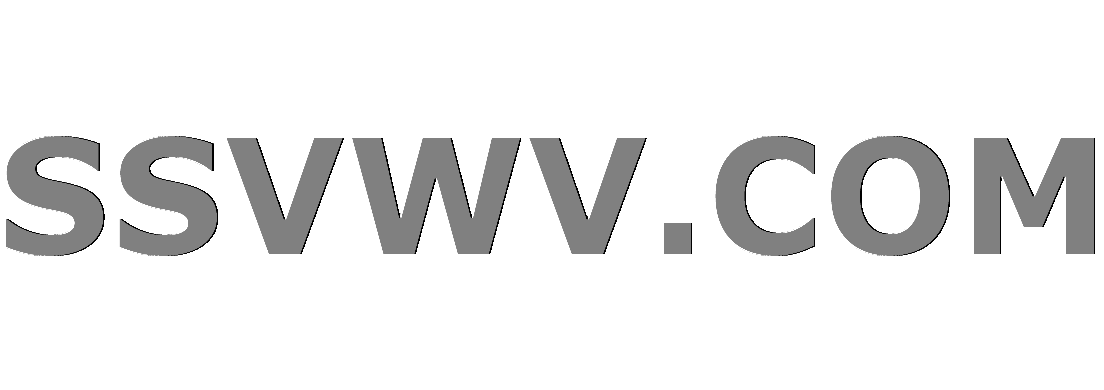
Multi tool use
unity c# - how to get values of list<object>
I have this json:
[
[
[
"Tel Aviv",
"Beersheba",
"Jerusalem",
"Haifa"
]
],
[
{
"City": "Tel Aviv"
},
{
"City": "Beersheba"
},
{
"City": "Jerusalem"
},
{
"City": "Haifa"
},
{
"City": "Jerusalem"
},
{
"City": "Tel Aviv"
},
{
"City": "Haifa"
},
{
"City": "Beersheba"
},
{
"City": "Jerusalem"
},
{
"City": "Jerusalem"
},
{
"City": "Haifa"
},
{
"City": "Tel Aviv"
},
{
"City": "Tel Aviv"
},
{
"City": "Beersheba"
}
]
]
And i converted it to a List:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Newtonsoft;
string jsonStr = "<json string from above>";
List<object> data;
private void Start()
{
data = Newtonsoft.Json.JsonConvert.DeserializeObject<List<object>>(jsonStr);
Debug.Log(data[0,0]);
}
But when the dubugger got to "Debug.Log(data[0,0]);" it printed:
Severity Code Description Project File Line Suppression State
Error CS0021 Cannot apply indexing with to an expression of type 'object' Assembly-CSharp C:Userscdi2DownloadsmdClone-20180627T083334Z-001mdCloneAssetsCreateTable.cs 31 Active
the first value: "Tel Aviv"
– raz erez
Jul 1 at 9:08
1 Answer
1
Your string is deserialized in a List<object>
A list can be indexed with only one dimension.
So the deserialization creates only two objects of type JArray.
The first one is the group of four cities and the second one is an array of 14 cities.
List<object>
You can get the first element of the first object with
Console.WriteLine((data[0] as JArray)[0][0]);
While, if you want to reach any of the elements in the second JArray you can use this syntax
Console.WriteLine((data[1] as JArray)[1]["City"]); // Beersheba
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
And what do you expect it to print?
– Steve
Jul 1 at 9:01