Iterate over every nth element in string in loop - python
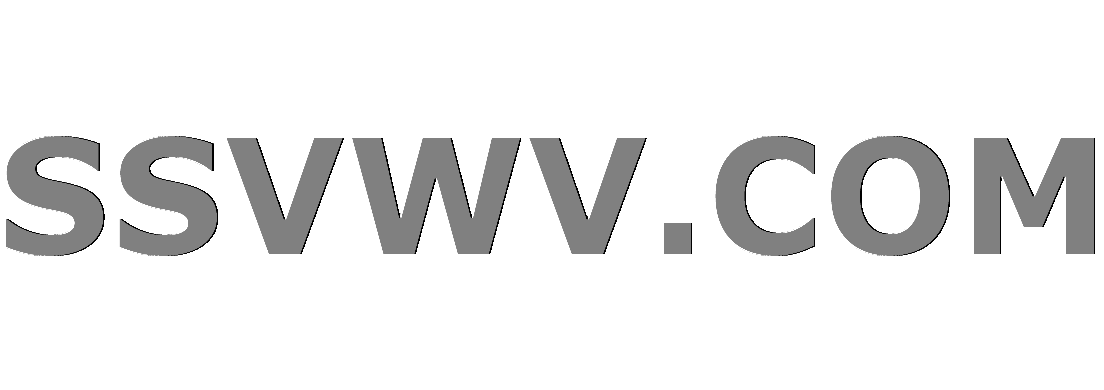
Multi tool use
Iterate over every nth element in string in loop - python
How can iterate over every second element in string ?
One way to do this would be(If I want to iterate over nth element):
sample = "This is a string"
n = 3 # I want to iterate over every third element
i = 1
for x in sample:
if i % n == 0:
# do something with x
else:
# do something else with x
i += 1
Is thery any "pythonic" way to do this? (I am pretty sure my method is not good)
for i,x in enumerate(sample,1):
3 Answers
3
If you want to do something every nth step, and something else for other cases, you could use enumerate
to get the index, and use modulus:
enumerate
sample = "This is a string"
n = 3 # I want to iterate over every third element
for i,x in enumerate(sample):
if i % n == 0:
print("do something with x "+x)
else:
print("do something else with x "+x)
Note that it doesn't start at 1 but 0. Add an offset to i
if you want something else.
i
To iterate on every nth element only, the best way is to use itertools.islice
to avoid creating a "hard" string just to iterate on it:
itertools.islice
import itertools
for s in itertools.islice(sample,None,None,n):
print(s)
result:
T
s
s
r
g
This is the most comprehensive answer
– Agile_Eagle
Jul 1 at 9:25
you can use step
for example sample[start:stop:step]
step
sample[start:stop:step]
If you want to iterate over every second element you can do :
sample = "This is a string"
for x in sample[::2]:
print(x)
output
T
i
s
a
s
r
n
That is a nice answer!!
– Agile_Eagle
Jul 1 at 9:12
it doesn't answer to "do something every even item and do something else every odd item" though.
– Jean-François Fabre
Jul 1 at 9:22
@Jean-FrançoisFabre Yes but still it is useful and partly answers my question
– Agile_Eagle
Jul 1 at 9:24
Try using slice
:
slice
sample = "This is a string"
for i in sample[slice(None,None,2)]:
print(i)
Output:
T
i
s
a
s
r
n
sample[slice(None,None,2)]
is the same as sample[::2]
. Using slice
in that context isn't really useful. It still creates a sub-list– Jean-François Fabre
Jul 1 at 9:19
sample[slice(None,None,2)]
sample[::2]
slice
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
for i,x in enumerate(sample,1):
– Jean-François Fabre
Jul 1 at 9:11