Codeigniter task scheduling
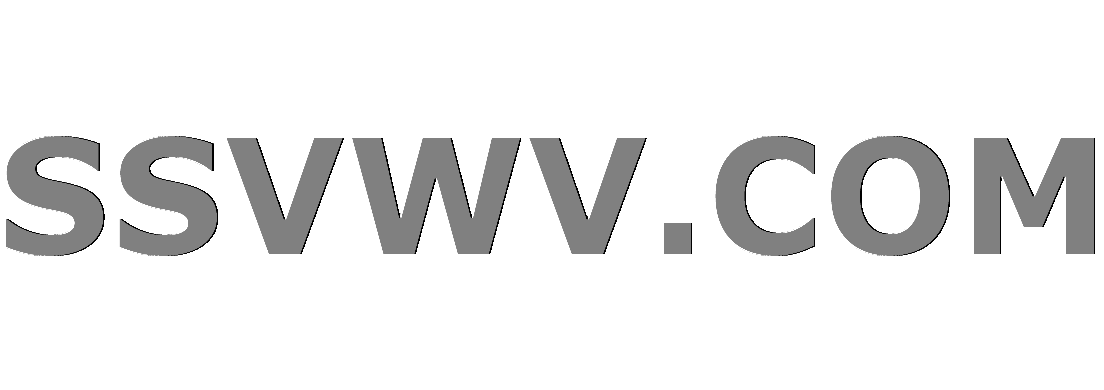
Multi tool use
Codeigniter task scheduling
I am not very much sure if my title question is correct, however i am facing sever error of multiple loops within foreach, despite of having only one item in array. I am pasting my code here;
Display (Controller)
$table_data = array(
'table_data' => $this->display_model->get_table_data($table_name),
'edit_table_data'=>$this->display_model->get_table_data($table_name,$row_id)
);
$form_name='edit_'.$table_name;
$this->load->view('header');
$this->load->view($form_name,$table_data);
$this->load->view('footer');
You can see tabe_data and edit_table_data is calling same function with different parameter.
Here i doubt on the time scheduling between these two function calls (which honestly i am wrong because codeigniter manages function calls)
Display_model (Model)
public function get_table_data($table_name,$row_id=null)
{
$return = "";
if ($row_id != null)
{
switch ($table_name) {
case 'user':
//$return = $this->db->where($table_name."_id",$row_id)->get($table_name)->result();
$return = $this->db->select($table_name.'.*,country.country_name')
->join('country','country.country_id=user.user_country_id','left')
->where($table_name.'_id',$row_id)
->get($table_name)->result();
break;
case 'user_document':
//$return = $this->db->where($table_name."_id",$row_id)->get($table_name)->result();
$return = $this->db->select($table_name.'.*,document.document_name')
->join('document','document.document_id=use_document.document_id','left')
->where($table_name.'_id',$row_id)
->get($table_name)->result();
break;
default:
$return = $this->db->where($table_name."_id",$row_id)->get($table_name)->result();
break;
}
if($return != null)
{
return $return;
}
else
{
return "no_data";
}
}
Here is my view where i am getting error
<?php foreach ($edit_table_data as $etd_row) {?>
<form method="post" class="form-horizontal" action="<?php echo site_url('admin/edit_row/user_type/'.$etd_row->user_type_id)?>">
<div class="form-group">
<label class="col-sm-4 control-label">User Type Name</label>
<div class="col-sm-8"><input type="text" class="form-control" name="user_type_name" value="<?php echo $etd_row->user_type_name?>"></div>
</div>
<div class="hr-line-dashed"></div>
<div class="form-group">
<div class="col-sm-2 col-sm-offset-2">
<button class="btn btn-primary" type="submit">Edit changes</button>
</div>
</div>
</form>
<?php } ?>
on the foreach loop line, i am given error
Thanks in advance for your comments
Error
A PHP Error was encountered
Severity: Warning
Message: Invalid argument supplied for foreach()
Filename: views/edit_user_type.php
Line Number: 18
Backtrace:
File: C:wampwwwgmfapplicationviewsedit_user_type.php
Line: 18
Function: _error_handler
File: C:wampwwwgmfapplicationcontrollersDisplay.php
Line: 170
Function: view
File: C:wampwwwgmfindex.php
Line: 315
Function: require_once
check for empty like this in your view
if (! empty($edit_table_data)){ foreach($edit_table_data as $etd_row){}}
– pradeep
Jul 1 at 8:08
if (! empty($edit_table_data)){ foreach($edit_table_data as $etd_row){}}
Actually $edit_table_data is an array and it is not null, did check this in model and view, both places
– Shariati
Jul 1 at 9:04
pls always response to the answers by giving some comments or ,if it helps you, by marking it as green and upvoting, it is the best way to thanks all the programmers
– pradeep
12 hours ago
3 Answers
3
You'd get this error if $edit_table_data
is not an array -- in this case probably a string.
$edit_table_data
My CI knowledge is a bit rusty, but I guess something along these lines may help you out:
$query = $this->db->select($table_name.'.*,country.country_name')
->join('country','country.country_id=user.user_country_id','left')
->where($table_name.'_id',$row_id)
->get($table_name);
if ($query->num_rows() === 0){
return ; // Make sure we're always returning an array.
// I'd rather handle this stuff in the view...
// Probably some kind of message that shows:
// that there are not results when $edit_table_data is not an array, otherwise loop.
}
return $query->result();
Or you could do something along these lines:
<?php
if (!is_array($edit_table_data)) {
echo 'No results here.';
} else {
foreach ($edit_table_data as $etd_row) { ?>
<form method="post" class="form-horizontal"
action="<?php echo site_url('admin/edit_row/user_type/' . $etd_row->user_type_id) ?>">
<div class="form-group">
<label class="col-sm-4 control-label">User Type Name</label>
<div class="col-sm-8"><input type="text" class="form-control" name="user_type_name"
value="<?php echo $etd_row->user_type_name ?>"></div>
</div>
<div class="hr-line-dashed"></div>
<div class="form-group">
<div class="col-sm-2 col-sm-offset-2">
<button class="btn btn-primary" type="submit">Edit changes</button>
</div>
</div>
</form>
<?php
}
}
?>
I have updated my question and added error, can you please check. Problem is that the array is not empty and it is an array because the edit for is being populated, meaning that foreach loop is run. However, it runs on an on without being stopped.
– Shariati
Jul 1 at 9:03
Hope this will help you :
Return records before break
in every case
like this :
break
case
public function get_table_data($table_name,$row_id=null)
{
if ($row_id != null)
{
switch ($table_name) {
case 'user':
$return = $this->db->select($table_name.'.*,country.country_name')
->join('country','country.country_id=user.user_country_id','left')
->where($table_name.'_id',$row_id)
->get($table_name)->result();
return $return;
break;
case 'user_document':
$return = $this->db->select($table_name.'.*,document.document_name')
->join('document','document.document_id=use_document.document_id','left')
->where($table_name.'_id',$row_id)
->get($table_name)->result();
return $return;
break;
default:
$return = $this->db->where($table_name."_id",$row_id)->get($table_name)->result();
return $return;
break;
}
}
Your view should be like this :
Note : its not a good idea to loop your form
within foreach
loop , keep form
outside of the loop
form
foreach
form
Check for empty $edit_table_data
in your form before the loop like this :
$edit_table_data
<?php if ( ! empty($edit_table_data)) {
foreach ($edit_table_data as $etd_row) {?>
<form method="post" class="form-horizontal" action="<?php echo site_url('admin/edit_row/user_type/'.$etd_row->user_type_id)?>">
<div class="form-group">
<label class="col-sm-4 control-label">User Type Name</label>
<div class="col-sm-8">
<input type="text" class="form-control" name="user_type_name" value="<?php echo $etd_row->user_type_name?>">
</div>
</div>
<div class="hr-line-dashed"></div>
<div class="form-group">
<div class="col-sm-2 col-sm-offset-2">
<button class="btn btn-primary" type="submit">Edit changes</button>
</div>
</div>
</form>
<?php }} ?>
Thanks @pradeep, I returned data in each case. Now, i am near to solution. what happens is foreach is looping indefinitely. How to stop this ?
– Shariati
Jul 1 at 11:39
you can check to stop it when it reaches some particular key or value within your loop
– pradeep
Jul 1 at 11:42
by the way if my answer helps you pls don't hesitate to upvote and check it as green
– pradeep
Jul 1 at 11:42
well, since i am looking to edit a particular record so i am pulling 1 record only, but it loops indefinitely
– Shariati
Jul 1 at 11:52
if you want to edit particular record first way, is use
row()
instead of result()
in your database query in switch
, second way , is add an anchor with href which has id of that particular record within your foreach loop, this way you can edit a record , secode one is much better– pradeep
Jul 1 at 12:03
row()
result()
switch
There does not seem to be a reason to call get_table_data
twice, once with and once without $row_id
, because you never use $table_data['$table_data']
in the view. In the interests of getting something that works that first call should be eliminated. The revised controller looks like this.
get_table_data
$row_id
$table_data['$table_data']
$table_data['edit_table_data'] = $this->display_model->get_table_data($table_name,$row_id) ;
$form_name='edit_'.$table_name;
$this->load->view('header');
$this->load->view($form_name,$table_data);
$this->load->view('footer');
The model code in the question is missing a curly brace }
to close this statement.
}
if ($row_id != null)
{
So it is a little hard to tell exactly what you want to happen if $row_id
is not provided. Without further information, my opinion is that get_table_data
should not give the second argument a default value. In other words, the function should be defined without a default value for `$row_id - like this.
$row_id
get_table_data
public function get_table_data($table_name, $row_id)
Without a default value for `$row_id a fatal error occurs if no value is provided. So the controller will need to make sure a value is provided.
Likewise, the controller should always check the return of the model is appropriate, or the model should always return something that the view can use.
get_table_data()
contains a lot of repeated code - where($table_name.'_id', $row_id)
and get($table_name)->result()
appear three times each. By rearranging the logic, the repeating lines can be eliminated to make the function much more concise. Consider this version.
get_table_data()
where($table_name.'_id', $row_id)
get($table_name)->result()
public function get_table_data($table_name, $row_id)
{
if($table_name === 'user')
{
$this->db
->select($table_name.'.*, country.country_name')
->join('country', 'country.country_id = user.user_country_id', 'left');
}
elseif($table_name === 'user_document')
{
$this->db
->select($table_name.'.* ,document.document_name')
->join('document', 'document.document_id = use_document.document_id', 'left');
}
return $this->db
->where($table_name."_id", $row_id)
->get($table_name)
->row();
}
The above returns data from any $table_name
where $table_name."_id" == $row_id
. Specific "select" and "join" clauses are added in the case of 'user' or 'user_document' tables.
$table_name
$table_name."_id" == $row_id
Because you seem to be interested in only one row you should call row()
instead of result()
.
row()
result()
It's important to know that db->row()
will return NULL if no records are found. That fact can be used to test the model return and react appropriately. In this case, we do the check in the view. Because there is only one row. no foreach
is needed.
db->row()
foreach
<?php if(isset($edit_table_data)) { ?>
<form method="post" class="form-horizontal" action="<?php echo site_url('admin/edit_row/user_type/'.$edit_table_data->user_type_id) ?>">
<div class="form-group">
<label class="col-sm-4 control-label">User Type Name</label>
<div class="col-sm-8"><input type="text" class="form-control" name="user_type_name" value="<?php echo $edit_table_data->user_type_name ?>"></div>
</div>
<div class="hr-line-dashed"></div>
<div class="form-group">
<div class="col-sm-2 col-sm-offset-2">
<button class="btn btn-primary" type="submit">Edit changes</button>
</div>
</div>
</form>
<?php
}
else { ?> <div>No Data Available</div> <?php } ?>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
get_table_data can return a string (no_data) which is an invalid argument for foreach. If this is the case, make it return an empty array instead of a string.
– dn Fer
Jul 1 at 7:58