How == and equal works in java in Case of Integer Object? [duplicate]
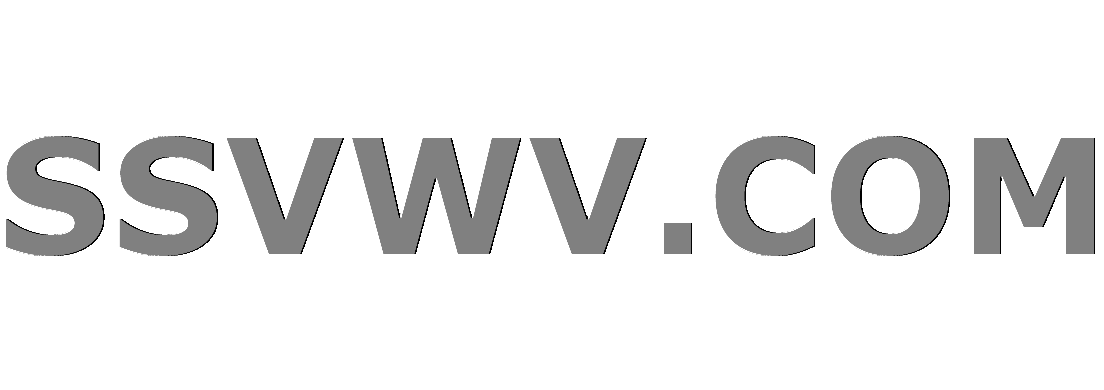
Multi tool use
How == and equal works in java in Case of Integer Object? [duplicate]
This question already has an answer here:
I have found many possible duplicates question on this but none clarifies my doubt on how it works?
Integer a =25654; // a.hashCode()=>25654
Integer b =25654; // b.hashCode()=>25654
System.out.println(a.equals(b)); => true
System.out.println(a == b); => false
I have read this answer somewhere..
If no parent classes have provided an override, then it defaults to the method from the ultimate parent class, Object, and so you're left with the Object#equals(Object o) method. Per the Object API this is the same as ==; that is, it returns true if and only if both variables refer to the same object, if their references are one and the same. Thus you will be testing for object equality and not functional equality.
in this case both object have same memory address(as per hashcode) still why does it returns false when we compare using == ? or the actual memory address is different? please correct me if i am wrong. Thanks
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
hashCode
equals
25654
a == b
false
is there a way to see the actual memory address of the Integer object?
– Sharan Rai
Jul 1 at 8:07
You should hardly ever care about the actual memory address of an object (and the JVM can move objects in memory!)
– Mark Rotteveel
Jul 1 at 8:10
Why do you think they're in the same memory address? These are two different objects. They can't possibly be at the same address.
– Dawood ibn Kareem
Jul 1 at 8:11
Reopened. A good duplicate would discuss integer caching. This part of java is very bewildering: flawed, almost.
– Bathsheba
Jul 1 at 8:17
2 Answers
2
Object.hashCode
:
Object.hashCode
The hashCode method defined by class Object does return distinct
integers for distinct objects.
But Integer.hashCode
overrides Object.hashCode
:
Integer.hashCode
Object.hashCode
returns a hash code value for this object, equal to the primitive int
value represented by this Integer object.
which means Integers with same hashCode does not have to share the same object.
The source code of Integer.hashCode
Integer.hashCode
@Override
public int hashCode() {
return Integer.hashCode(value);
}
public static int hashCode(int value) {
return value;
}
If you let:
Integer a = 127;
Integer b = 127;
System.out.println(a.equals(b)); // true
System.out.println(a == b); // true
this is because Integers between [-128, 127] could be cached.
in this case both object have same memory address(as per hashcode)
still why does it returns false when we compare using == ? or the
actual memory address is different? please correct me if i am wrong.
Thanks
The hashcode has not to be considered as the memory address because the internal address of an object may change through the time.
The specification of hashCode()
never state that two objects with the same hashcode value refer necessarily the same object.
Even two objects with the same hashcode may not be equals
in terms of Object.equals()
.
hashCode()
equals
Object.equals()
But the specification states :
If two objects are equal according to the equals(Object) method, then
calling the hashCode method on each of the two objects must produce
the same integer result.
And this is verifiable in your example :
Integer a =25654; // a.hashCode()=>25654
Integer b =25654; // a.hashCode()=>25654
System.out.println(a.equals(b)); => true
a
and b
refer to objects that are equals()
, and yes their hash code is indeed the same.
a
b
equals()
Your quotation doesn't refer to hashCode()
but to the default Object.equals()
method that will be used if no overriding was done for equals()
.
hashCode()
Object.equals()
equals()
For example if I don't override equals() and hashCode() for a Foo class :
Foo fooOne = new Foo(1);
Foo fooOneBis = new Foo(1);
fooOne.equals(fooOneBis); // return false
The two objects have the same state but they are not equals as under the hood is used Object.equals()
that compares the object itself and not their state.
Object.equals()
And indeed equal()
will return true only if the variables refer the same object :
equal()
Foo foo = new Foo(1);
foo.equals(foo); // return true
Integer class itself overrides both
hashCode
andequals
method. HashCode simply returns value held by Integer object, in this case25654
despite the fact that both objects are different instances (which you can see viaa == b
->false
).– Pshemo
Jul 1 at 7:58