Replacing maximum value with minimum value in a array [on hold]
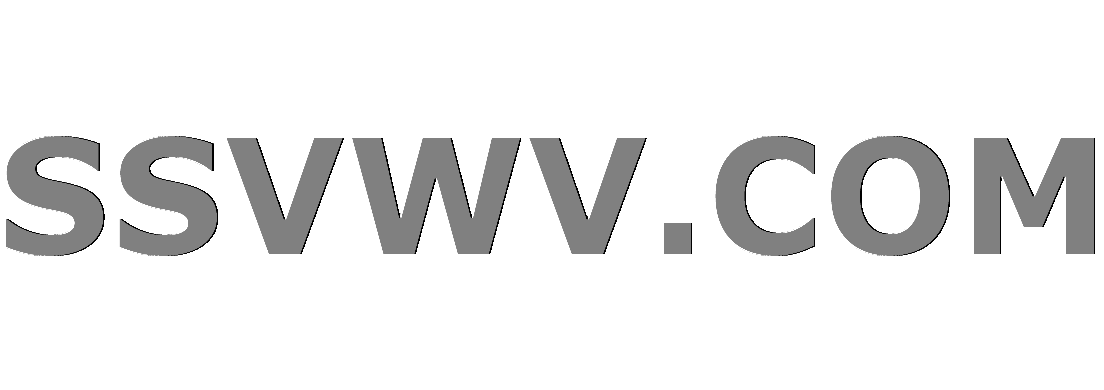
Multi tool use
Replacing maximum value with minimum value in a array [on hold]
First input is n numbers then the numbers itself, then find the maximum value and minimum value and last, replace the maximum value with the minimum one. But this code doesn't work first test case ( 5 1 3 3 3 4 ), it returns 3 3 3 3 3. But it works on second test case ( 8 5 4 2 2 4 2 2 5 ). What is wrong with this code ? I have checked the loop which check for maximum and minimum value, nothing wrong.
import java.util.Scanner;
class Main {
public static void main(String args){
Scanner sc = new Scanner(System.in);
int marks = new int[sc.nextInt()];
int max = 0;
int min = 100;
for(int i=0;i<marks.length;i++){
marks[i] = sc.nextInt();
}
for(int i=0;i<marks.length;i++){
if(min>marks[i]){
min = marks[i];
}
}
for(int i=0;i<marks.length;i++){
if(max<marks[i]){
max = marks[i];
}
}
for(int i=0;i<marks.length;i++){
if(marks[i]==max){
marks[i]=min;
}
}
for(int i=0;i<marks.length;i++){
if(i!=marks.length-1){
System.out.print(marks[i]+" ");
}else{
System.out.println(marks[i]);
System.out.println();
}
}
}
}
This question appears to be off-topic. The users who voted to close gave these specific reasons:
Hey this works fine for me.
– anoopknr
Jul 1 at 6:50
Code works fine. See IDEONE.
– Andreas
Jul 1 at 7:04
Ok so it's a problem from my machine. I'm using Sublime text by the way. Thank you so much !
– Andrean Lay
Jul 1 at 7:14
2 Answers
2
I think your code should work fine. I refactored it to make it more robust, readable and to make it easier to fire the test cases in a reproducable way:
public static void main(String args) {
int first = new int{1, 3, 3, 3, 4};
replaceMaxWithMin(first);
System.out.println("******************************************");
int second = new int{5, 4, 2, 2, 4, 2, 2, 5};
replaceMaxWithMin(second);
System.out.println("******************************************");
int fromScanner = readFromScanner();
System.out.println("From scanner: " + Arrays.toString(fromScanner));
replaceMaxWithMin(fromScanner);
}
private static void replaceMaxWithMin(int marks) {
int max = Integer.MIN_VALUE;
int min = Integer.MAX_VALUE;
for (int mark : marks) {
min = Math.min(min, mark);
max = Math.max(max, mark);
}
for (int i = 0; i < marks.length; i++) {
if (marks[i] == max) {
marks[i] = min;
}
}
for (int i = 0; i < marks.length; i++) {
if (i != marks.length - 1) {
System.out.print(marks[i] + " ");
} else {
System.out.println(marks[i]);
System.out.println();
}
}
}
private static int readFromScanner() {
Scanner sc = new Scanner(System.in);
int marks = new int[sc.nextInt()];
for(int i=0;i<marks.length;i++){
marks[i] = sc.nextInt();
}
return marks;
}
The output for a run on my Java 8 JVM:
1 3 3 3 1
******************************************
2 4 2 2 4 2 2 2
******************************************
5 1 3 3 3 4 <--- what I typed into the console
From scanner: [1, 3, 3, 3, 4]
1 3 3 3 1
So the replacement code itelf works fine. Probably you have some weird problems with your entered data. You could also println
the array before running your algorithm with Arrays.toString(marks);
. At least for me, everything including reading from the scanner works fine.
println
Arrays.toString(marks);
Good luck!
Bro, I just cross-checked your code and found that atint marks = new int[sc.nextInt()]
it will take only one integer and so the size of the array will be the first integer entered. So, to overcome through that you should predefine the size of the array OR you can take ArrayList
for more convenience.
int marks = new int[sc.nextInt()]
ArrayList
And, a little suggestion from my side is whenever you want to find the maximum or minimum from the elements of the array you can set the minimum and maximum variable as
min = arr[0];
max = arr[0];
for(int i=0;i<n;i++){
if(min>arr[i])
min = arr[i];
if(max<arr[i])
max = arr[i];
}
This will help you. Happy coding :)
Sorry, but your first sentence is just wrong. It reads the first entered input (the 5 or 8) and initializes the array with that size.
– Marcus K.
Jul 1 at 8:04
Better. But still no need for "you should predefine the size of the array OR" because that is exactly what the questioner does ;-) And yes, I would also prefer an ArrayList for this so you don't need to enter the amount of numbers as first input :-)
– Marcus K.
Jul 1 at 8:11
ok, but I have mentioned that for more user convenience as the user will not have to change the array again and again in the code :)
– Asheesh Sahu
Jul 1 at 8:15
There's no
n
defined in the OP's code and your code will fail, if the number of elements the user inputs is 0
. It would be preferable to use Integer.MAX_VALUE
and Integer.MIN_VALUE
as initial values for min
and max
respectively.– fabian
Jul 2 at 14:01
n
0
Integer.MAX_VALUE
Integer.MIN_VALUE
min
max
Your first test case returns 1 3 3 3 1 when run on my machine.
– Sweeper
Jul 1 at 6:49