how do I fix the type mismatch issue?
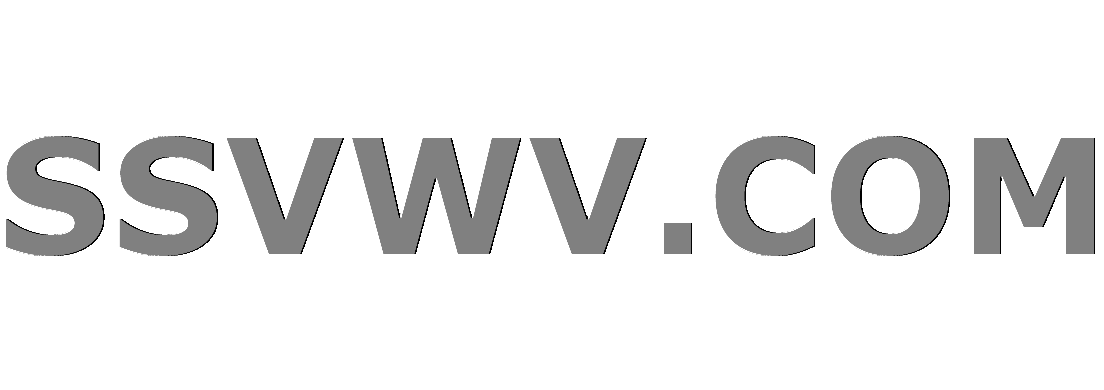
Multi tool use
how do I fix the type mismatch issue?
I have a spark streaming job and had a question about type converting. the below is my code:
val component = data.get("viewed_objects").get.asInstanceOf[ListBuffer[Map[String, Any]]]
but the exception is
scala.collection.immutable.$colon$colon cannot be cast to scala.collection.ListBuffer
what caused the issue and how do I fix it?
I print the data value, it is for example: data:Map(viewed_objects -> List(Map(location -> 2, category_name -> Toys, Kids & Babies, category_id -> 27))), how do I get the viewed_objects and traversal the elements
– Zhang Xin
Jul 1 at 3:52
I would get the viewed_objects list and deal with every element in the list
– Zhang Xin
Jul 1 at 4:03
And you don't get that error for the data you have given in the comments. you get the error when you do
.asInstanceOf[Map[String, Any]]
. So you are just mixing code and errors. Please test it again and update with the right code and right error message and of course sample data as well– Ramesh Maharjan
Jul 1 at 4:06
.asInstanceOf[Map[String, Any]]
yeah, you are right, I had fixed it ,thank you so much
– Zhang Xin
Jul 1 at 4:28
1 Answer
1
In scala scala.collection.immutable.$colon$colon
simply means that the data is scala.collection.immutable.List
since List
are the generated using cons
(::
) notation.
scala.collection.immutable.$colon$colon
scala.collection.immutable.List
List
cons
::
So the error
scala.collection.immutable.$colon$colon cannot be cast to scala.collection.ListBuffer
means that you are trying to convert List
to ListBuffer
and they are not compatible.
List
ListBuffer
So what you can do is change ListBuffer
to List
as
ListBuffer
List
val component = data.get("viewed_objects").get.asInstanceOf[List[Map[String, Any]]]
but that would be useless type casting as data.get("viewed_objects").get
is already of List[Map[String, Any]]
type
data.get("viewed_objects").get
List[Map[String, Any]]
so just doing
val component = data.get("viewed_objects").get
//component: List[scala.collection.immutable.Map[String,Any]] = ...
would be enough
I hope the answer is helpful
Update
You have commented as
btw, if data value is data:Map(), that means an empty Map, your code would return exception None.get exception. How to handle it gracefully?
for that you use Try
getOrElse
as
Try
getOrElse
val component = Try(data.get("viewed_objects").get).getOrElse(List(Map.empty[String, Any]))
Or you can use pattern matching as
val component = data.get("viewed_objects") match {case Some(data) => data; case None => List(Map.empty[String, Any])}
btw, if data value is data:Map(), that means an empty Map, your code would return exception None.get exception. How to handle it gracefully?
– Zhang Xin
Jul 1 at 4:56
thank you, I use your codes, its type is scala.collection.immutable.$colon$colon, I want to traverse it, using for (element <- component), but it doesn't work. how to traverse it or convert to List type?
– Zhang Xin
Jul 1 at 7:54
how to read the elements of component
– Zhang Xin
Jul 1 at 7:57
the issue was because the try or pattern matching was returning Any . now that I have modifed the answer to return List[Map[String, Any]] from both. the for or foreach should work
– Ramesh Maharjan
Jul 1 at 8:03
yeah,I have, it does work, thank you
– Zhang Xin
Jul 1 at 8:24
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
yes,it is a json String,like this: u'data': {u'viewed_objects': [{u'location': 3, u'keyword': u'baby carriers'}]}
– Zhang Xin
Jul 1 at 3:47