How to include navigation property without direct access to foreign key ID using Entity Framework?
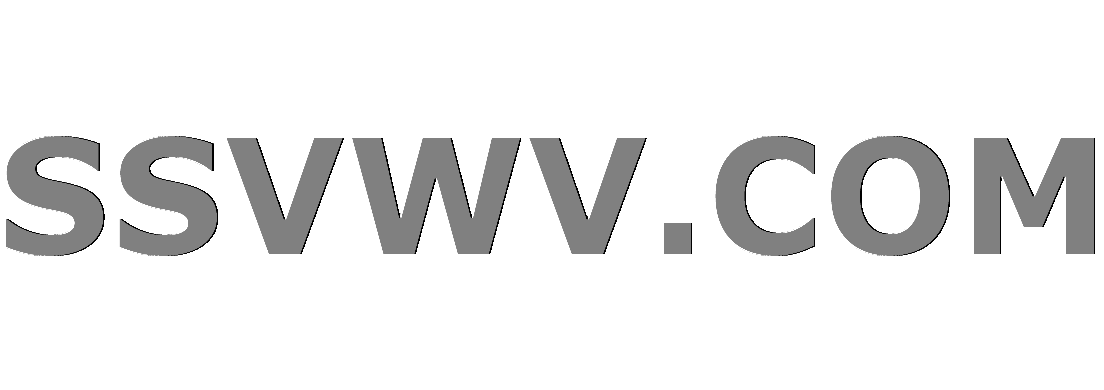
Multi tool use
How to include navigation property without direct access to foreign key ID using Entity Framework?
I have two tables set up as one-to-many with the "one" model being Artist
:
Artist
public class Artist
{
public Artist()
{
this.Albums = new HashSet<Album>();
}
public int ArtistID { get; set; }
public string UserID { get; set; }
[Display(Name = "Artist name")]
public string ArtistName { get; set; }
public virtual ICollection<Album> Albums { get; set; }
}
and the "many" being Album
:
Album
public class Album
{
public int AlbumID { get; set; }
public int ArtistID { get; set; }
public string StringExt { get; set; }
public virtual Artist Artist { get; set; }
}
Now on an album page load, my url uses an id of type string called ID
(for example https://website.com/to/albumName) to load the album page. Here's the controller:
ID
// GET: To
public ActionResult Index(string ID)
{
if (String.IsNullOrWhiteSpace(ID))
{
return RedirectToAction("Index", "Home");
}
var model = new ToViewModel();
model.Album = EntityDataAccess.GetAlbumByStringExt(ID);
model.Artist = model.Album.Artist;
return View(model);
}
BUT I need to include the Artist
class on page load, so logically I'd like to use:
Artist
public static Album GetAlbumByStringExt(string stringExt)
{
using (var Context = GetContext())
{
return Context.Albums.Include("Artist").Where(x => x.StringExt == stringExt).FirstOrDefault().;
}
}
But since the foreign key for Artists
is the int
value of ArtistID
it does not get included in my database query since I am searching with stringExt
.
Artists
int
ArtistID
stringExt
My first thought was to query through the db without the include("Artist")
, grab ahold of the Album object
, and then query AGAIN using the ArtistID
that I now have from the new Album
object. This doesn't seem very efficient, so I was wondering if there is a better method of doing this?
include("Artist")
Album object
ArtistID
Album
Thanks!
Context.Albums.Include(a => a.Artist).FirstOrDefault(x => x.StringExt == stringExt);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Entity framework doesn't need the FK when the model is properly configured (looks right to me).
Context.Albums.Include(a => a.Artist).FirstOrDefault(x => x.StringExt == stringExt);
should retrieve the matching album and its artist. If it does not, insure they exist in the database and examine the SQL.– Steve Greene
Jul 1 at 15:28