Parsing dynamic json in go
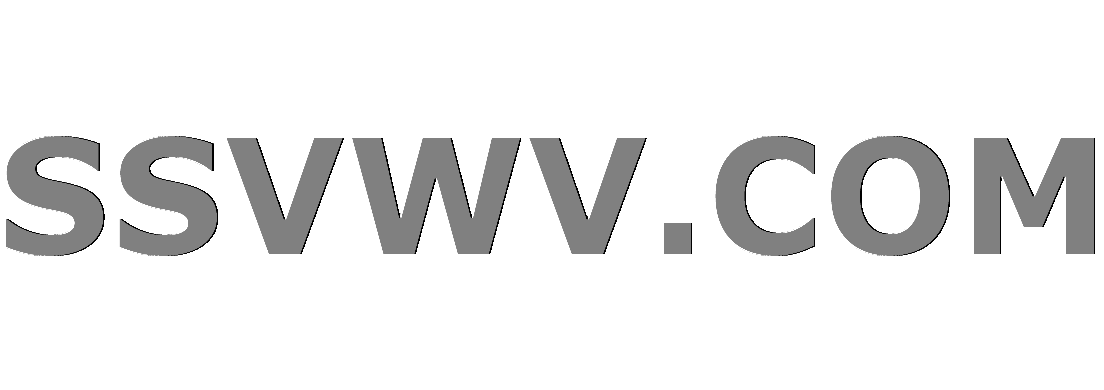
Multi tool use
Parsing dynamic json in go
I am trying to parse the following json structure, where the fields marked with "val1" and "val2" are constantly changing, so I cannot use a predefined struct. How could I parse this json in a way to be able to loop through every single "val"? Thank you!
{"result":true,"info":{"funds":{"borrow":{"val1":"0","val2":"0"},"free":{"val1":"0","val2":"0"},"freezed":{"val1":"0","val2":"0"}}}}
struct { Borrow, Free, Freezed map[string]interface{} }
Possible duplicate of stackoverflow.com/questions/44011905/parse-dynamic-json-object
– ThunderCat
Jun 30 at 21:31
2 Answers
2
By unmarshalling into the following struct I can loop through the desired fields.
type Fields struct {
Result bool `json:"result"`
Info struct {
Funds struct {
Borrow, Free, Freezed map[string]interface{}
} `json:"funds"`
} `json:"info"`
}
package main
import (
"fmt"
"encoding/json"
)
type Root struct {
Result bool `json:"result"`
Info Info `json:"info"`
}
type Info struct {
Funds struct {
Borrow, Free, Freezed map[string]interface{}
} `json:"funds"`
}
func main() {
var rootObject Root
jsonContent := " {"result":true,"info":{"funds":{"borrow":{"val1":"0","val2":"0"},"free":{"val1":"0","val2":"0"},"freezed":{"val1":"0","val2":"0"}}}}"
if err := json.Unmarshal(byte(jsonContent), &rootObject); err != nil {
panic(err)
}
fmt.Println(rootObject)
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
struct { Borrow, Free, Freezed map[string]interface{} }
– ThunderCat
Jun 30 at 20:09