Translating a C++ line to Python 3
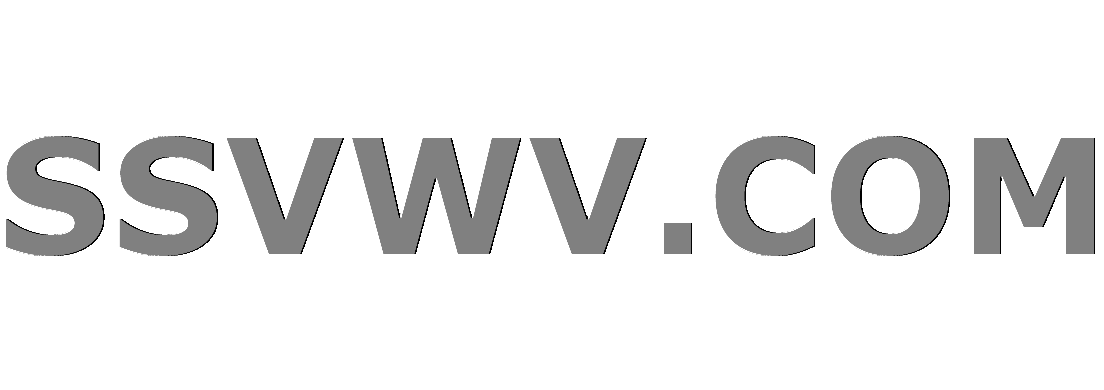
Multi tool use
Translating a C++ line to Python 3
I'm trying to translate a Qt plugin from C++ to python, however my knowledge on C++ is minimal, can you please help me to confirm if my translation is Ok? (the plugin is not working as expected).
C++ code:
/***************************************************************************
* Copyright (C) 2006-2008 by Tomasz Ziobrowski *
* http://www.3electrons.com *
* e-mail: t.ziobrowski@3electrons.com *
* *
* *
* This program is free software; you can redistribute it and/or modify *
* it under the terms of the GNU General Public License as published by *
* the Free Software Foundation; either version 2 of the License, or *
* (at your option) any later version. *
* *
* This program is distributed in the hope that it will be useful, *
* but WITHOUT ANY WARRANTY; without even the implied warranty of *
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the *
* GNU General Public License for more details. *
* *
* You should have received a copy of the GNU General Public License *
* along with this program; if not, write to the *
* Free Software Foundation, Inc., *
* 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA. *
***************************************************************************/
#include <QPainter>
#include <QPen>
#include <QSvgRenderer>
#include "counter.h"
Counter::Counter (QWidget * parent):QWidget(parent)
{
init();
}
int Counter::digits() const
{
return m_digits;
}
int Counter::value() const
{
return m_value;
}
const QString Counter::digitsFile() const
{
return m_digitsFile;
}
/*--------------------------------------------------------------------------------------------
P U B L I C S L O T S
--------------------------------------------------------------------------------------------*/
void Counter::setValue(int i)
{
m_value = i;
update();
}
void Counter::setDigits(int i)
{
m_digits = i;
update();
}
void Counter::setDigitsFile(const QString & i )
{
m_digitsFile = i;
if (m_svg)
delete m_svg;
m_svg = new QSvgRenderer(this);
if (!m_svg->load(m_digitsFile))
{
qDebug("Counter::setDigitsFile can't load file %s",qPrintable(m_digitsFile));
m_digitsFile = ":/default/resources/train_digits.svg";
m_svg->load(m_digitsFile);
}
else
qDebug("Counter::setDigitsFile %s loaded",qPrintable(m_digitsFile));
update();
}
/*--------------------------------------------------------------------------------------------
P R O T E C T E D
--------------------------------------------------------------------------------------------*/
#define X_OFFSET 10
#define Y_OFFSET 10
void Counter::paintEvent (QPaintEvent *)
{
QPainter p(this);
p.setRenderHint(QPainter::Antialiasing,true);
p.save();
int side = this->height();
p.scale(side/100.0,side/100.0);
double width = 100.0 * this->width()/this->height(), height = 100.0;
//p.setPen(Qt::NoPen);
QPen pen = p.pen();
pen.setColor(QColor(32,32,32));
pen.setWidthF(6.0);
p.setPen(pen);
p.setBrush(Qt::black);
p.drawRoundRect(3,3,width-6,height-6,7,(7*width)/(double)height);
int w = (width-2*X_OFFSET)/m_digits;
int x = (m_digits-1) * w;
int h = height-2*Y_OFFSET;
int r, c = m_value;
for (int i=0 ; i<m_digits ; i++)
{
r = c % 10;
c = c/10;
QRect rect(x+X_OFFSET,Y_OFFSET,w,h);
m_svg->render(&p,QString("d%1").arg(r),rect);
x -= w;
}
p.restore();
}
void Counter::init()
{
Q_INIT_RESOURCE(analogwidgets);
m_digits = 4;
m_value = 0;
m_svg = NULL;
setDigitsFile(":/default/resources/train_digits.svg");
}
My Python translation:
from PyQt5.QtGui import QPainter, QPen, QColor
from PyQt5.QtSvg import QSvgRenderer
from PyQt5.QtCore import *
from PyQt5.QtWidgets import QWidget
import sys
import os
class PyCounter(QWidget):
def __init__(self, parent = None):
super(PyCounter, self).__init__(parent)
self.inicio()
def getDigits(self):
return self.m_digits
def getValue(self):
return self.m_value
def getDigitsfile(self):
return self.m_digitsFile
def setValue(self, val):
self.m_value = val
self.update()
def setDigits(self, dit):
self.m_digits = dit
self.update()
def setDigitsFile(self, filemon):
self.m_digitsFile = filemon
if self.m_svg == True:
del self.m_svg
self.m_svg = QSvgRenderer(self)
if self.m_svg.load(self.m_digitsFile) == False:
print("Cannot load resource File")
self.m_digitsFile = ":/default/resources/train_digits.svg"
self.m_svg.load(self.m_digitsFile)
else:
print("Train digits correctly loaded")
self.update()
X_OFFSET = 10
Y_OFFSET = 10
def paintEvent(self, event):
p = QPainter(self)
p.setRenderHint(QPainter.Antialiasing)
p.save()
side = self.height()
p.scale(side/100.0, side/100.0)
width = 100 * self.width()
height = 100 * self.height()
pen = QPen(p.pen())
pen.setColor(QColor(32,32,32))
pen.setWidthF(6.0)
p.setPen(pen)
p.setBrush(Qt.black)
p.drawRoundedRect(3,3,width-6,height-6,7,(7*width)/height)
w = (width - 2*(10))/self.m_digits
x = (self.m_digits-1) * w
h = height-2*10
c = self.m_value
r = 0
y = 0
while y < self.m_digits:
y += 1
r = c % 10
c = c/10
rect = QRectF(x + 10, 10, w, h)
self.m_svg.render(p, str("d%1").format(r), rect)
x -= w
p.restore()
def inicio(self):
import AnalogWidgets_rc
self.m_value = 0
self.m_digits = 4
self.m_svg = None
self.setDigitsFile(":/default/resources/train_digits.svg")
My doubt is specifically on line 69 of the C++ code, where it says:
m_svg = new QSvgRenderer(this);
I don't know exactly how to translate this, that "new" make me feel I'm doing it wrong, check my translation:
self.m_svg = QSvgRenderer(self)
What is not working as expected is the SVG load.
EDIT:
This is how the widgets looks like:
PyCounter
AnalogWidgets_rc.py:
https://pastebin.com/Ck0VX6eG
That file was generated using the pyrcc5 tool
Original analogwidgets.qrc:
<RCC>
<qresource prefix="/default" >
<file>resources/train_digits.svg</file>
<file>resources/dial1.svg</file>
<file>resources/dial2.svg</file>
<file>resources/dial3.svg</file>
<file>resources/dial4.svg</file>
</qresource>
</RCC>
self.m_svg = QSvgRenderer()
Yes, same result, background painted, no SVG loaded.
– Ronald Petit
31 mins ago
Can you check if
self.m_svg.load(self.m_digitsFile)
returns True
? That would at least mean that the file was actually loaded. Then you would need to call render()
– konoufo
27 mins ago
self.m_svg.load(self.m_digitsFile)
True
render()
It does, if I change the "False" to "True" I get the output of the if statement on console (the print funcion saying cannot load resource file). Maybe I need to do something else with the resource file I'm importing?
– Ronald Petit
25 mins ago
@RonaldPetit see my answer :)
– eyllanesc
8 mins ago
1 Answer
1
I see that the problem is in the following line:
self.m_svg.render(p, str("d%1").format(r), rect)
%1
is used by QString to complete data, in the case of python when using format you must use {}
:
%1
{}
self.m_svg.render(p, "d{}".format(r), rect)
In the following link you can find the complete code.
Maybe that was an error, however it makes no difference, now I really feel the problem is the file I'm importing, as I removed the line "import AnalogWidgets_rc" and makes no difference, I will attach that file and also post a picture of how the widgets looks like
– Ronald Petit
5 mins ago
@RonaldPetit Have you downloaded my code?
– eyllanesc
1 min ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Have you tried with just
self.m_svg = QSvgRenderer()
?– konoufo
33 mins ago