Why does this Rust binary tree overflow its stack in tests? [duplicate]
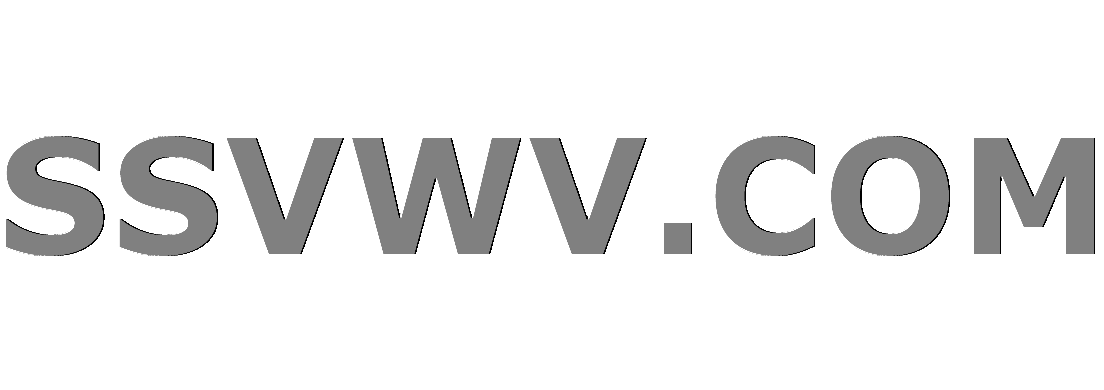
Multi tool use
Why does this Rust binary tree overflow its stack in tests? [duplicate]
This question already has an answer here:
I've written a binary tree-like structure, but I've been getting stack-overflow errors in some of my stress tests. I've reduced the error-causing code down to the following:
struct Node {
index: usize,
right: Option>,
}
struct BoxedTree {
root: Option<Box<Node>>,
}
fn build_degenerate() {
let mut t = BoxedTree {
root: Some(Box::new(Node {
index: 0,
right: None,
})),
};
let mut n = t.root.as_mut().unwrap();
for i in 1..50000 {
let cur = n;
let p = &mut cur.right;
*p = Some(Box::new(Node {
index: i,
right: None,
}));
n = p.as_mut().unwrap();
}
println!("{}", n.index);
}
fn main() {
build_degenerate();
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn mytest() {
build_degenerate();
}
}
Running cargo test
gives me:
cargo test
running 1 test
thread 'tests::mytest' has overflowed its stack
fatal runtime error: stack overflow
error: process didn't exit successfully: ...
I don't understand how this code could be causing a stack overflow, as all data seems to be allocated on the heap, and there is no recursion.
Even stranger, this code causes an overflow when I run cargo test
, but not when I run cargo run
, even though mytest
and main
call the same code.
cargo test
cargo run
mytest
main
What's going wrong here?
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
TL;DR: "and there is no recursion" is a lie and tests have a smaller stack size.
– Shepmaster
Jul 1 at 3:20
Ah, so the default destructor is recursive; that makes perfect sense, thank you.
– Isaac
Jul 1 at 4:15
I believe your question is answered by the answers of Do we need to manually create a destructor for a linked list?, Why does my iterative implementation of drop for a linked list still cause a stack overflow? and cargo test --release causes a stack overflow. Why doesn't cargo bench?. If you disagree, please edit your question to explain the differences. Otherwise, we can mark this question as already answered.
– Shepmaster
Jul 1 at 3:20