Viewing Variables of Another Python File Without Importing/Running
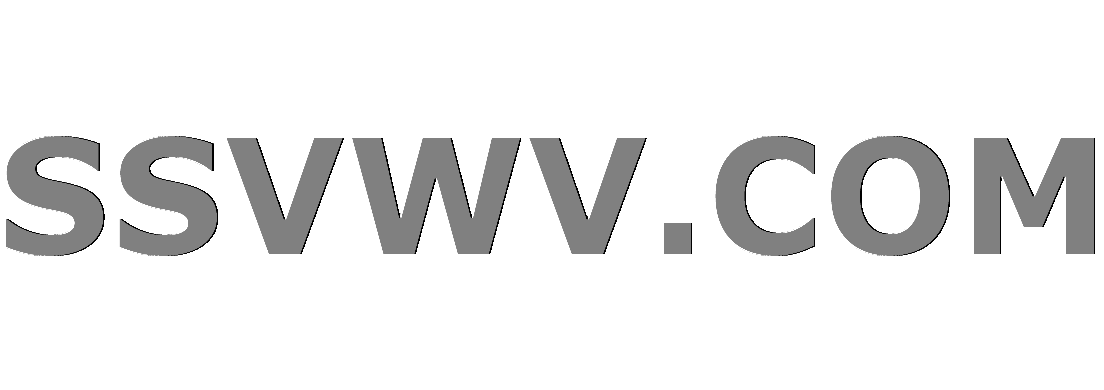
Multi tool use
Viewing Variables of Another Python File Without Importing/Running
I have been trying to read python files and print its variables for a while now. Is it possible to view and print the variables of another file without importing or running it? Everything I have already searched only explains how to import or use execfile, etc... (disclaimer, I am probably dumb)
This is what I have so far:
for vars in dir():
print(vars)
Now, this works perfectly fine for the file it is being run from, but when I try this code:
for vars in file:
print(vars)
(file is simply path.read())
Well, it gives me every character of the file on a new line. I have no idea if my loop is correct at all. Will I have to write something that will manually find each variable, then add it into a list?
what do you mean by "variable"?
– Azat Ibrakov
Jun 30 at 20:01
please, provide an example of module you want to read "variables" from and desired output
– Azat Ibrakov
Jun 30 at 20:02
I mean something simple like variable = "hello"
– Kaigott
Jun 30 at 20:03
You could compile it and then disassemble it. See
compile
and dis
– Peter Wood
Jun 30 at 20:08
compile
dis
3 Answers
3
Use ast.parse
to parse the code, recursively traverse the nodes by iterating through those with a body
attribute (which are code blocks), look for Assign
objects and get their targets
(which are the variables being assigned with values, which are what you're looking for) and get their id
attribute if they are Name
objects.
ast.parse
body
Assign
targets
id
Name
Try the following code after replacing file.py
with the file name of the python script you want to parse.
file.py
import ast
import _ast
def get_variables(node):
variables = set()
if hasattr(node, 'body'):
for subnode in node.body:
variables |= get_variables(subnode)
elif isinstance(node, _ast.Assign):
for name in node.targets:
if isinstance(name, _ast.Name):
variables.add(name.id)
return variables
print(get_variables(ast.parse(open('file.py').read())))
Thanks, it works great! The docs are really confusing. Can you explain a little more what this does?
– Kaigott
Jul 1 at 3:59
Glad to be of help. Yeah the docs of
ast
and compile
aren't very thorough at all. I had to dump these node objects myself to learn their data structures. I think I've already explained my logic above the code though. Is there a specific part of the code that you can't see what it's doing? Basically the idea is, since you are only looking for variable assignments, I only look for Assign
nodes within the code blocks, and put the assignment targets into a set as the returning value.– blhsing
Jul 1 at 4:05
ast
compile
Assign
Nothing very specific, just wanted clarification on what
node
does– Kaigott
Jul 1 at 4:33
node
No ... and yes.
The question is whether the "variables" are constants or true variables.
Python runs a garbage collector. It will create the variables when you run/import a module. These variables will have scope based on how they are used. Once they are no longer in use, the garbage collector will remove the objects from memory.
If the variable is assigned a fixed value (e.g. i = 1
) then you can simply read the file in as if it is a text file - since it is a text file. If you want to change those variables, you can simply write it out as a text file. It will be incumbent on you to trace the variables in the text, exactly as with any text matching.
i = 1
If the variables are generated as part of the code, then no (e.g. it generates a list of file in a directory and assigns to a variable). You would need to either import the module, or change the module so that it exports the outputs to a separate file - e.g. csv - and then you can read the data file.
First of all you are not dumb, its just not many people need to do this.
Also, I cant think of a way without moving the variables to a septate json or pickle file then loading it in later in both programs using json.load(filename)
or something like that. But that only works if it isn't being changed while the other program is assessing it.
json.load(filename)
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You can take some inspiration from Python source file parsers: stackoverflow.com/questions/768634/…
– Vlad Frolov
Jun 30 at 19:51