Decreasing a static image progress bar horizontally in SDL
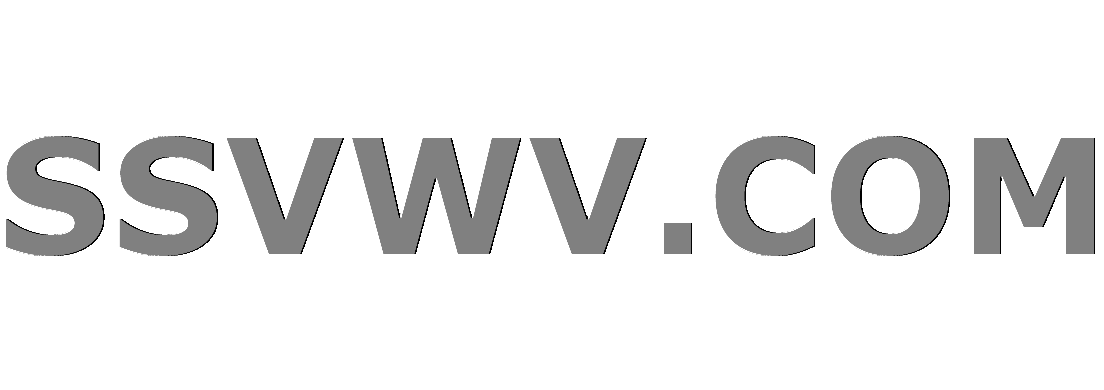
Multi tool use
Decreasing a static image progress bar horizontally in SDL
In my project, I have a decreasing timer progress bar PNG image on display. This image and its background can be found at the below link:
https://s3.eksiup.com/df7dd38f781.png
My goal is to make the bar decrease starting from the upper green side to the lower red side. My code for this is the following:
void EntityTimer::OnRender(SDL_Renderer *ren)
{
SDL_Rect currRect,dstRect;
double rem=0.0;
memcpy(&currRect,&origRect,sizeof (SDL_Rect));
memcpy(&dstRect,&origRect,sizeof (SDL_Rect));
if (timerObjPtr->remainingDuration>timerObjPtr->durationInMilisec)
timerObjPtr->durationInMilisec=timerObjPtr->remainingDuration;
rem=((double)timerObjPtr->remainingDuration/timerObjPtr->durationInMilisec);
currRect.h=(origRect.h)*rem;
currRect.h = currRect.h;
dstRect.x=0;
dstRect.y=0;
dstRect.h=currRect.h;
SDL_RenderCopy(ren,timerTexture,&dstRect,&currRect);
}
Since this image is static, I tried to do it by manipulating this texture's height parameter with "currRect.h=(origRect.h)*rem;" line. But this makes the progress bar to decrease from the red side to the green side (the opposite of what I want).
I tried to correct it but made it worse by mirroring the PNG image on the progress bar area and decreasing it from bottom to top again.
Any help is appreciated so that the bar decreases from top (green) to bottom (red).
1 Answer
1
Well that's kinda expected since you're drawing from the same point (x = 0, y = 0) but using a smaller size. You need to update the destine y position:
dstRect.y = origRect.h - origRect.h * rem;
EDIT: It was the other way around
dstRect.y = origRect.h * rem
(assuming rem goes from 0 to 1)
Here is an example of what you want, you can use this image to test https://i.imgur.com/faKKShU.png
#include <SDL2/SDL.h>
#include <SDL2/SDL_image.h>
#include <iostream>
#include <thread>
#define HEIGHT 600
#define WIDTH 800
using namespace std;
int main() {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window *window = SDL_CreateWindow("Red", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, WIDTH, HEIGHT, SDL_WINDOW_SHOWN);
SDL_Renderer *renderer = SDL_CreateRenderer(window, 0, SDL_RENDERER_ACCELERATED | SDL_RENDERER_PRESENTVSYNC);
bool quit = false;
SDL_Event event;
SDL_Texture *red_part = IMG_LoadTexture(renderer, "red_image.png");
float multiplier = 0;
while (!quit) {
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
quit = true;
}
}
int y_pos = HEIGHT * multiplier;
SDL_RenderClear(renderer);
SDL_Rect copy_rect{0, y_pos, 800, 600};
SDL_RenderCopy(renderer, red_part, nullptr, ©_rect);
SDL_RenderPresent(renderer);
multiplier += 0.01;
if (multiplier >= 1.)
multiplier = 1.;
std::this_thread::sleep_for(std::chrono::milliseconds{33});
}
SDL_DestroyWindow(window);
SDL_DestroyRenderer(renderer);
SDL_Quit();
return 0;
}
@semihthor Can you post a MVCE?
– Aram
Jun 29 at 15:34
@semihthor I had my math the other way around since the screen actually increases to the bottom and to the right. Updated with a working example.
– Aram
Jun 30 at 14:42
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I tried adding your suggestion to my code but it isn't doing what I really want it to do. The result of your suggestion is below: gph.is/2KskE7Y After seeing your suggestion, I changed my code as following: currRect.y = origRect.h - (origRect.h) * rem; dstRect.y = currRect.y; below is its result: gph.is/2NbwUeG I achieved decreasing the bar from green to red but the position is still problematic.
– semihthor
Jun 29 at 6:02