Getting visitors country from their IP
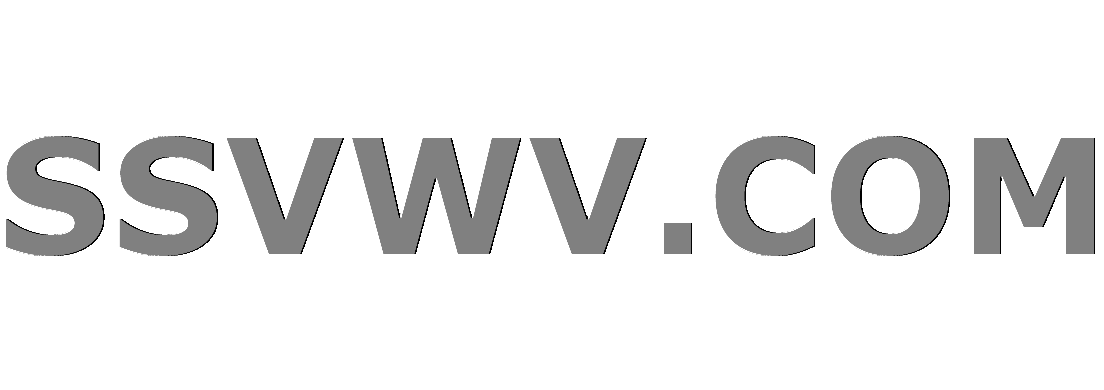
Multi tool use
Getting visitors country from their IP
I want to get visitors country via their IP... Right now I'm using this (http://api.hostip.info/country.php?ip=...... )
Here is my code:
<?php
if (isset($_SERVER['HTTP_CLIENT_IP']))
{
$real_ip_adress = $_SERVER['HTTP_CLIENT_IP'];
}
if (isset($_SERVER['HTTP_X_FORWARDED_FOR']))
{
$real_ip_adress = $_SERVER['HTTP_X_FORWARDED_FOR'];
}
else
{
$real_ip_adress = $_SERVER['REMOTE_ADDR'];
}
$cip = $real_ip_adress;
$iptolocation = 'http://api.hostip.info/country.php?ip=' . $cip;
$creatorlocation = file_get_contents($iptolocation);
?>
Well, it's working properly, but the thing is, this returns the country code like US or CA., and not the whole country name like United States or Canada.
So, is there any good alternative to hostip.info offers this?
I know that I can just write some code that will eventually turn this two letters to whole country name, but I'm just too lazy to write a code that contains all countries...
P.S: For some reason I don't want to use any ready made CSV file or any code that will grab this information for me, something like ip2country ready made code and CSV.
Use Maxmind geoip feature. It will include the country name in the results. maxmind.com/app/php
– Tchoupi
Sep 23 '12 at 14:36
Your first assignment to
$real_ip_address
is always ignored. Anyways, remember that the X-Forwarded-For
HTTP header can be extremely easily counterfeited, and that there are proxies like www.hidemyass.com– Walter Tross
Mar 29 '14 at 23:02
$real_ip_address
X-Forwarded-For
hostip.info seems to no longer be available try ipdata.co
– Jonathan
Sep 5 '17 at 17:57
IPLocate.io provides a free API:
https://www.iplocate.io/api/lookup/8.8.8.8
- Disclaimer: I run this service.– ttarik
Nov 15 '17 at 0:47
https://www.iplocate.io/api/lookup/8.8.8.8
20 Answers
20
Try this simple PHP function.
<?php
function ip_info($ip = NULL, $purpose = "location", $deep_detect = TRUE) {
$output = NULL;
if (filter_var($ip, FILTER_VALIDATE_IP) === FALSE) {
$ip = $_SERVER["REMOTE_ADDR"];
if ($deep_detect) {
if (filter_var(@$_SERVER['HTTP_X_FORWARDED_FOR'], FILTER_VALIDATE_IP))
$ip = $_SERVER['HTTP_X_FORWARDED_FOR'];
if (filter_var(@$_SERVER['HTTP_CLIENT_IP'], FILTER_VALIDATE_IP))
$ip = $_SERVER['HTTP_CLIENT_IP'];
}
}
$purpose = str_replace(array("name", "n", "t", " ", "-", "_"), NULL, strtolower(trim($purpose)));
$support = array("country", "countrycode", "state", "region", "city", "location", "address");
$continents = array(
"AF" => "Africa",
"AN" => "Antarctica",
"AS" => "Asia",
"EU" => "Europe",
"OC" => "Australia (Oceania)",
"NA" => "North America",
"SA" => "South America"
);
if (filter_var($ip, FILTER_VALIDATE_IP) && in_array($purpose, $support)) {
$ipdat = @json_decode(file_get_contents("http://www.geoplugin.net/json.gp?ip=" . $ip));
if (@strlen(trim($ipdat->geoplugin_countryCode)) == 2) {
switch ($purpose) {
case "location":
$output = array(
"city" => @$ipdat->geoplugin_city,
"state" => @$ipdat->geoplugin_regionName,
"country" => @$ipdat->geoplugin_countryName,
"country_code" => @$ipdat->geoplugin_countryCode,
"continent" => @$continents[strtoupper($ipdat->geoplugin_continentCode)],
"continent_code" => @$ipdat->geoplugin_continentCode
);
break;
case "address":
$address = array($ipdat->geoplugin_countryName);
if (@strlen($ipdat->geoplugin_regionName) >= 1)
$address = $ipdat->geoplugin_regionName;
if (@strlen($ipdat->geoplugin_city) >= 1)
$address = $ipdat->geoplugin_city;
$output = implode(", ", array_reverse($address));
break;
case "city":
$output = @$ipdat->geoplugin_city;
break;
case "state":
$output = @$ipdat->geoplugin_regionName;
break;
case "region":
$output = @$ipdat->geoplugin_regionName;
break;
case "country":
$output = @$ipdat->geoplugin_countryName;
break;
case "countrycode":
$output = @$ipdat->geoplugin_countryCode;
break;
}
}
}
return $output;
}
?>
How to use:
Example1: Get visitor IP address details
<?php
echo ip_info("Visitor", "Country"); // India
echo ip_info("Visitor", "Country Code"); // IN
echo ip_info("Visitor", "State"); // Andhra Pradesh
echo ip_info("Visitor", "City"); // Proddatur
echo ip_info("Visitor", "Address"); // Proddatur, Andhra Pradesh, India
print_r(ip_info("Visitor", "Location")); // Array ( [city] => Proddatur [state] => Andhra Pradesh [country] => India [country_code] => IN [continent] => Asia [continent_code] => AS )
?>
Example 2: Get details of any IP address. [Support IPV4 & IPV6]
<?php
echo ip_info("173.252.110.27", "Country"); // United States
echo ip_info("173.252.110.27", "Country Code"); // US
echo ip_info("173.252.110.27", "State"); // California
echo ip_info("173.252.110.27", "City"); // Menlo Park
echo ip_info("173.252.110.27", "Address"); // Menlo Park, California, United States
print_r(ip_info("173.252.110.27", "Location")); // Array ( [city] => Menlo Park [state] => California [country] => United States [country_code] => US [continent] => North America [continent_code] => NA )
?>
why im getting unknow all the time with every ip ? , used same code.
– echo_Me
Feb 18 '14 at 18:12
You get "Unknown" probably because your server does not allow
file_get_contents()
. Just check your error_log file. Workaround: see my answer.– Kai Noack
Feb 19 '14 at 12:47
file_get_contents()
also it might be because u check it localy (192.168.1.1 / 127.0.0.1 / 10.0.0.1)
– Hontoni
May 2 '14 at 13:38
@EchtEinfachTV I can not get the country name as well, when I echo $cip, $iptolocation and $creatorlocation I get this: 10.48.44.43http://api.hostip.info/country.php?ip=10.48.44.43XX , why it returns xx for country?
– mOna
Sep 21 '14 at 16:04
@Antonimo: I can not get the country name as well, when I echo $cip, $iptolocation and $creatorlocation I get this: 10.48.44.43http://api.hostip.info/country.php?ip=10.48.44.43XX , why it returns xx for country? –
– mOna
Sep 21 '14 at 16:05
You can use a simple API from http://www.geoplugin.net/
$xml = simplexml_load_file("http://www.geoplugin.net/xml.gp?ip=".getRealIpAddr());
echo $xml->geoplugin_countryName ;
echo "<pre>";
foreach ($xml as $key => $value)
{
echo $key , "= " , $value , " n" ;
}
echo "</pre>";
Function Used
function getRealIpAddr()
{
if (!empty($_SERVER['HTTP_CLIENT_IP'])) //check ip from share internet
{
$ip=$_SERVER['HTTP_CLIENT_IP'];
}
elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) //to check ip is pass from proxy
{
$ip=$_SERVER['HTTP_X_FORWARDED_FOR'];
}
else
{
$ip=$_SERVER['REMOTE_ADDR'];
}
return $ip;
}
Output
United States
geoplugin_city= San Antonio
geoplugin_region= TX
geoplugin_areaCode= 210
geoplugin_dmaCode= 641
geoplugin_countryCode= US
geoplugin_countryName= United States
geoplugin_continentCode= NA
geoplugin_latitude= 29.488899230957
geoplugin_longitude= -98.398696899414
geoplugin_regionCode= TX
geoplugin_regionName= Texas
geoplugin_currencyCode= USD
geoplugin_currencySymbol= $
geoplugin_currencyConverter= 1
It makes you have so many options you can play around with
Thanks
:)
That's really cool. But while testing here are no values in the following fields "geoplugin_city, geoplugin_region, geoplugin_regionCode, geoplugin_regionName".. What is the reason? Is there any solution? Thanks in advance
– WebDevRon
Feb 28 '15 at 20:50
And how do you get just one value e.g "geoplugin_countryCode" ???
– Precious Tom
Nov 3 '17 at 15:53
I tried Chandra's answer but my server configuration does not allow file_get_contents()
PHP Warning: file_get_contents() URL file-access is disabled in the server configuration
PHP Warning: file_get_contents() URL file-access is disabled in the server configuration
I modified Chandra's code so that it also works for servers like that using cURL:
function ip_visitor_country()
{
$client = @$_SERVER['HTTP_CLIENT_IP'];
$forward = @$_SERVER['HTTP_X_FORWARDED_FOR'];
$remote = $_SERVER['REMOTE_ADDR'];
$country = "Unknown";
if(filter_var($client, FILTER_VALIDATE_IP))
{
$ip = $client;
}
elseif(filter_var($forward, FILTER_VALIDATE_IP))
{
$ip = $forward;
}
else
{
$ip = $remote;
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "http://www.geoplugin.net/json.gp?ip=".$ip);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
$ip_data_in = curl_exec($ch); // string
curl_close($ch);
$ip_data = json_decode($ip_data_in,true);
$ip_data = str_replace('"', '"', $ip_data); // for PHP 5.2 see stackoverflow.com/questions/3110487/
if($ip_data && $ip_data['geoplugin_countryName'] != null) {
$country = $ip_data['geoplugin_countryName'];
}
return 'IP: '.$ip.' # Country: '.$country;
}
echo ip_visitor_country(); // output Coutry name
?>
Hope that helps ;-)
Actually, you can call http://api.hostip.info/?ip=123.125.114.144 to get the information, which is presented in XML.
api.hostip.info doesn't resolve try api.ipdata.co/123.125.114.144
– Jonathan
Oct 25 '17 at 16:14
Use MaxMind GeoIP (or GeoIPLite if you are not ready to pay).
$gi = geoip_open('GeoIP.dat', GEOIP_MEMORY_CACHE);
$country = geoip_country_code_by_addr($gi, $_SERVER['REMOTE_ADDR']);
geoip_close($gi);
@Joyce: I tried to use Maxmind API and DB, but I don't know why it does not work for me, actually it works in general, but for instance when I run this $_SERVER['REMOTE_ADDR'];it shows me this ip : 10.48.44.43, but when i use it in geoip_country_code_by_addr($gi, $ip), it returns nothing, any idea?
– mOna
Sep 21 '14 at 15:13
It is a reserved ip address (internal ip address from your local network). Try running the code on a remote server.
– Joyce Babu
Sep 21 '14 at 15:27
Use following services
1) http://api.hostip.info/get_html.php?ip=12.215.42.19
2)
$json = file_get_contents('http://freegeoip.appspot.com/json/66.102.13.106');
$expression = json_decode($json);
print_r($expression);
3) http://ipinfodb.com/ip_location_api.php
Check out php-ip-2-country from code.google. The database they provide is updated daily, so it is not necessary to connect to an outside server for the check if you host your own SQL server. So using the code you would only have to type:
<?php
$ip = $_SERVER['REMOTE_ADDR'];
if(!empty($ip)){
require('./phpip2country.class.php');
/**
* Newest data (SQL) avaliable on project website
* @link http://code.google.com/p/php-ip-2-country/
*/
$dbConfigArray = array(
'host' => 'localhost', //example host name
'port' => 3306, //3306 -default mysql port number
'dbName' => 'ip_to_country', //example db name
'dbUserName' => 'ip_to_country', //example user name
'dbUserPassword' => 'QrDB9Y8CKMdLDH8Q', //example user password
'tableName' => 'ip_to_country', //example table name
);
$phpIp2Country = new phpIp2Country($ip,$dbConfigArray);
$country = $phpIp2Country->getInfo(IP_COUNTRY_NAME);
echo $country;
?>
Example Code (from the resource)
<?
require('phpip2country.class.php');
$dbConfigArray = array(
'host' => 'localhost', //example host name
'port' => 3306, //3306 -default mysql port number
'dbName' => 'ip_to_country', //example db name
'dbUserName' => 'ip_to_country', //example user name
'dbUserPassword' => 'QrDB9Y8CKMdLDH8Q', //example user password
'tableName' => 'ip_to_country', //example table name
);
$phpIp2Country = new phpIp2Country('213.180.138.148',$dbConfigArray);
print_r($phpIp2Country->getInfo(IP_INFO));
?>
Output
Array
(
[IP_FROM] => 3585376256
[IP_TO] => 3585384447
[REGISTRY] => RIPE
[ASSIGNED] => 948758400
[CTRY] => PL
[CNTRY] => POL
[COUNTRY] => POLAND
[IP_STR] => 213.180.138.148
[IP_VALUE] => 3585378964
[IP_FROM_STR] => 127.255.255.255
[IP_TO_STR] => 127.255.255.255
)
we must provide database information to work ? seems not good.
– echo_Me
Feb 15 '14 at 22:49
We can use geobytes.com to get the location using user IP address
$user_ip = getIP();
$meta_tags = get_meta_tags('http://www.geobytes.com/IPLocator.htm?GetLocation&template=php3.txt&IPAddress=' . $user_ip);
echo '<pre>';
print_r($meta_tags);
it will return data like this
Array(
[known] => true
[locationcode] => USCALANG
[fips104] => US
[iso2] => US
[iso3] => USA
[ison] => 840
[internet] => US
[countryid] => 254
[country] => United States
[regionid] => 126
[region] => California
[regioncode] => CA
[adm1code] =>
[cityid] => 7275
[city] => Los Angeles
[latitude] => 34.0452
[longitude] => -118.2840
[timezone] => -08:00
[certainty] => 53
[mapbytesremaining] => Free
)
Function to get user IP
function getIP(){
if (isset($_SERVER["HTTP_X_FORWARDED_FOR"])){
$pattern = "/^(([1-9]?[0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5]).){3}([1-9]?[0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5])$/";
if(preg_match($pattern, $_SERVER["HTTP_X_FORWARDED_FOR"])){
$userIP = $_SERVER["HTTP_X_FORWARDED_FOR"];
}else{
$userIP = $_SERVER["REMOTE_ADDR"];
}
}
else{
$userIP = $_SERVER["REMOTE_ADDR"];
}
return $userIP;
}
I tried your code, it returns this for me: Array ( [known] => false )
– mOna
Sep 20 '14 at 16:06
when I try this : $ip = $_SERVER["REMOTE_ADDR"]; echo $ip; it returns it : 10.48.44.43, do you know what is the problem?? I used alspo maxmind geoip, and when I used geoip_country_name_by_addr($gi, $ip) againit returned me nothing...
– mOna
Sep 20 '14 at 16:09
@mOna, it return your ip address. for more details pls, share your code.
– Ram Sharma
Sep 22 '14 at 5:51
I found that problem is realted to my ip since it is for private network. then I fuond my real ip in ifconfig and used it in my program. then it worked:) Now, my question is how to get real ip in case of those users similar to me? (if they use local ip).. I wrote my code here: stackoverflow.com/questions/25958564/…
– mOna
Sep 22 '14 at 10:15
Try this simple one line code, You will get country and city of visitors from their ip remote address.
$tags = get_meta_tags('http://www.geobytes.com/IpLocator.htm?GetLocation&template=php3.txt&IpAddress=' . $_SERVER['REMOTE_ADDR']);
echo $tags['country'];
echo $tags['city'];
There is a well-maintained flat-file version of the ip->country database maintained by the Perl community at CPAN
Access to those files does not require a dataserver, and the data itself is roughly 515k
Higemaru has written a PHP wrapper to talk to that data: php-ip-country-fast
You can use a web-service from http://ip-api.com
in your php code, do as follow :
<?php
$ip = $_REQUEST['REMOTE_ADDR']; // the IP address to query
$query = @unserialize(file_get_contents('http://ip-api.com/php/'.$ip));
if($query && $query['status'] == 'success') {
echo 'Hello visitor from '.$query['country'].', '.$query['city'].'!';
} else {
echo 'Unable to get location';
}
?>
the query have many other informations:
array (
'status' => 'success',
'country' => 'COUNTRY',
'countryCode' => 'COUNTRY CODE',
'region' => 'REGION CODE',
'regionName' => 'REGION NAME',
'city' => 'CITY',
'zip' => ZIP CODE,
'lat' => LATITUDE,
'lon' => LONGITUDE,
'timezone' => 'TIME ZONE',
'isp' => 'ISP NAME',
'org' => 'ORGANIZATION NAME',
'as' => 'AS NUMBER / NAME',
'query' => 'IP ADDRESS USED FOR QUERY',
)
Used ip-api.com because they also supply ISP name!
– Richard Tinkler
May 11 '16 at 13:30
I Used because they also supply the Timezone
– Roy Shoa
Sep 10 '17 at 15:38
Many different ways to do it...
Solution #1:
One third party service you could use is http://ipinfodb.com. They provide hostname, geolocation and additional information.
Register for an API key here: http://ipinfodb.com/register.php. This will allow you to retrieve results from their server, without this it will not work.
Copy and past the following PHP code:
$ipaddress = $_SERVER['REMOTE_ADDR'];
$api_key = 'YOUR_API_KEY_HERE';
$data = file_get_contents("http://api.ipinfodb.com/v3/ip-city/?key=$api_key&ip=$ipaddress&format=json");
$data = json_decode($data);
$country = $data['Country'];
Downside:
Quoting from their website:
Our free API is using IP2Location Lite version which provides lower
accuracy.
Solution #2:
This function will return country name using the http://www.netip.de/ service.
$ipaddress = $_SERVER['REMOTE_ADDR'];
function geoCheckIP($ip)
{
$response=@file_get_contents('http://www.netip.de/search?query='.$ip);
$patterns=array();
$patterns["country"] = '#Country: (.*?) #i';
$ipInfo=array();
foreach ($patterns as $key => $pattern)
{
$ipInfo[$key] = preg_match($pattern,$response,$value) && !empty($value[1]) ? $value[1] : 'not found';
}
return $ipInfo;
}
print_r(geoCheckIP($ipaddress));
Output:
Array ( [country] => DE - Germany ) // Full Country Name
Quoting from their website: "You are limited to 1,000 API requests per day. If you need to make more requests, or need SSL support, see our paid plans."
– Walter Tross
Jul 10 '14 at 8:05
I used it on my personal website so that is why I posted it. Thank you for the info... didn't realize it. I put much more effort on the post, so please see the updated post :)
– imbondbaby
Jul 10 '14 at 9:42
@imbondbaby: Hi, I tried your code, but for me it returns this :Array ( [country] => - ), I do not understand the problem since when i try to print this: $ipaddress = $_SERVER['REMOTE_ADDR']; it shows me this ip : 10.48.44.43, I can't understand why this ip does not work! I mean wherever I insert this number it does not return any country!!! counld u plz help me?
– mOna
Sep 21 '14 at 14:46
Not sure if this is a new service but now (2016) the easiest way in php is to use geoplugin's php web service: http://www.geoplugin.net/php.gp:
Basic usage:
// GET IP ADDRESS
if (!empty($_SERVER['HTTP_CLIENT_IP'])) {
$ip = $_SERVER['HTTP_CLIENT_IP'];
} else if (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) {
$ip = $_SERVER['HTTP_X_FORWARDED_FOR'];
} else if (!empty($_SERVER['REMOTE_ADDR'])) {
$ip = $_SERVER['REMOTE_ADDR'];
} else {
$ip = false;
}
// CALL THE WEBSERVICE
$ip_info = unserialize(file_get_contents('http://www.geoplugin.net/php.gp?ip='.$ip));
They also provide a ready built class: http://www.geoplugin.com/_media/webservices/geoplugin.class.php.tgz?id=webservices%3Aphp&cache=cache
You used an
else
after else
which causes an error. What did you try to prevent? REMOTE_ADDR should be available all the time?– AlexioVay
Oct 27 '16 at 7:45
else
else
@Vaia - Perhaps it should be but you never know.
– billynoah
Nov 14 '16 at 19:59
Is there a case where it isnt available you know of?
– AlexioVay
Nov 14 '16 at 20:04
@Vaia - from the PHP docs on
$_SERVER
: "There is no guarantee that every web server will provide any of these; servers may omit some, or provide others not listed here."– billynoah
Nov 14 '16 at 20:08
$_SERVER
My service https://ipdata.co provides the country name in 5 languages! As well as the organisation, currency, timezone, calling code, flag, Carrier data, Proxy data and Tor Exit Node status data from any IPv4 or IPv6 address.
It's also extremely scalable with 10 regions around the world each able to handle >800M calls daily!
The options include; English (en), German (de), Japanese (ja), French (fr) and Simplified Chinese (za-CH)
$ip = '74.125.230.195';
$details = json_decode(file_get_contents("https://api.ipdata.co/{$ip}"));
echo $details->country_name;
//United States
echo $details->city;
//Mountain View
$details = json_decode(file_get_contents("https://api.ipdata.co/{$ip}/zh-CN"));
echo $details->country_name;
//美国
God bless you, man! I got more than what I asked for! Quick question: can I use it for prod? I mean, you're not going to put it down anytime soon, are you?
– Sayed
Nov 22 '17 at 19:25
Not at all :) I'm in fact adding more regions and more polish. Glad you found this to be of help :)
– Jonathan
Nov 22 '17 at 19:27
Very helpful, specially with the additional params, solved more than one problem for me!
– Sayed
Nov 22 '17 at 19:29
Thanks for the positive feedback! I built around the most common usecases for such a tool, the goal was to eliminate having to do any additional processing after the geolocation, happy to see this paying off for users
– Jonathan
Nov 22 '17 at 19:32
I have a short answer for this question which I have used in a project.
In my answer I have consider that you have visitor IP address
$ip = "202.142.178.220";
$ipdat = @json_decode(file_get_contents("http://www.geoplugin.net/json.gp?ip=" . $ip));
//get ISO2 country code
if(property_exists($ipdat, 'geoplugin_countryCode')) {
echo $ipdat->geoplugin_countryCode;
}
//get country full name
if(property_exists($ipdat, 'geoplugin_countryName')) {
echo $ipdat->geoplugin_countryName;
}
If it helps you vote up my answer.
I am using ipinfodb.com
api and getting exactly what you are looking for.
ipinfodb.com
Its completely free, you just need to register with them to get your api key. You can include their php class by downloading from their website or you can use url format to retrieve information.
Here's what I am doing:
I included their php class in my script and using the below code:
$ipLite = new ip2location_lite;
$ipLite->setKey('your_api_key');
if(!$_COOKIE["visitorCity"]){ //I am using cookie to store information
$visitorCity = $ipLite->getCity($_SERVER['REMOTE_ADDR']);
if ($visitorCity['statusCode'] == 'OK') {
$data = base64_encode(serialize($visitorCity));
setcookie("visitorCity", $data, time()+3600*24*7); //set cookie for 1 week
}
}
$visitorCity = unserialize(base64_decode($_COOKIE["visitorCity"]));
echo $visitorCity['countryName'].' Region'.$visitorCity['regionName'];
Thats it.
you can use http://ipinfo.io/ to get details of ip address Its easy to use .
<?php
function ip_details($ip)
{
$json = file_get_contents("http://ipinfo.io/{$ip}");
$details = json_decode($json);
return $details;
}
$details = ip_details(YoUR IP ADDRESS);
echo $details->city;
echo "<br>".$details->country;
echo "<br>".$details->org;
echo "<br>".$details->hostname; /
?>
Replace 127.0.0.1
with visitors IpAddress.
127.0.0.1
$country = geoip_country_name_by_name('127.0.0.1');
Installation instructions are here, and read this to know how to obtain City, State, Country, Longitude, Latitude, etc...
Please provide more actual code than just hard links.
– Bram Vanroy
May 9 '17 at 11:19
The User Country API has exactly what you need. Here is a sample code using file_get_contents() as you originally do:
$result = json_decode(file_get_contents('http://usercountry.com/v1.0/json/'.$cip), true);
$result['country']['name']; // this contains what you need
This API allows 100 (free) API calls per day.
– reformed
May 21 at 16:40
A one liner with an IP address to country API
echo file_get_contents('https://ipapi.co/8.8.8.8/country_name/');
> United States
Example :
https://ipapi.co/country_name/ - your country
https://ipapi.co/8.8.8.8/country_name/ - country for IP 8.8.8.8
This API allows 1000 (free) API calls per day.
– reformed
May 21 at 16:40
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
Don't be lazy, there aren't that many countries, and it's not too hard to obtain a translation table for FIPS 2 letter codes to country names.
– Chris Henry
Sep 23 '12 at 14:35