Give the player some friction when moving after being pushed
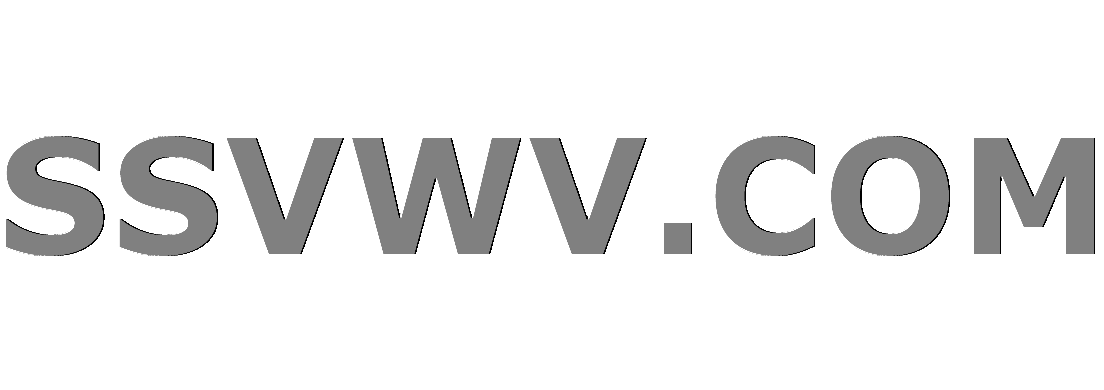
Multi tool use
Give the player some friction when moving after being pushed
Not really sure how to word this but lets say you are a ball and are bouncing around and moving. Its a flat surface so everything moves fine.
Now introduce some 45 degree ramps. You fall on one straight down and bounce away from it. At the moment you can instantly move back regardless of how soon you hit it or what angle or speed your traveling at where it should be harder from the momentum of the push because it was at an angle. Kind of like a small gradual momentum until you reach 0 velocity, then you can move normally.
if (playerLeft)
{
float xVelocity = (playerBody.velocity.x > 0) ? playerBody.velocity.x - playerSpeed : -playerSpeed;
playerBody.velocity = new Vector3(xVelocity, playerBody.velocity.y, 0);
}
if (playerRight)
{
float xVelocity = (playerBody.velocity.x < 0) ? playerBody.velocity.x + playerSpeed : playerSpeed;
playerBody.velocity = new Vector3(xVelocity, playerBody.velocity.y, 0);
}
Originally i only had 1 line for each where i would set the x velocity equal to the player speed regardless if the velocity was -1 or -50. You can imagine how unrealistic that looks.
I tried adding the velocity which is what i did above. But because its minimum is only ever around 6 below 0. It will reach its max speed in 2 or 3 frames which isnt gradual enough.
1 Answer
1
This is done with Physic Material.
1.First, create a a Physic Material by going to Assets ---> Create ---> Physic Material.
2.Drag the Physic Material to the Material slot of the Object's collider.
3.Below is what the Physics Material looks like when selected:
You can select the Physics Material and modify it's settings in the Editor or you can do that via script:
SphereCollider yourCollider = ...;
yourCollider.material.dynamicFriction = 0.6f;
yourCollider.material.staticFriction = 0.6f;
yourCollider.material.bounciness = 0.0f;
This allows you to modify the bounciness and friction of the object during run-time. I suggest you watch this video to understand the basic settings.
You may want to replace
playerBody.velocity
with playerBody.AddForce
then use the Physic Material example I provide in my answer yo change the friction. I suggest you read the doc to see the meaning of value. Also, I suggest you experiment while the game is running through the Editor and come up with proper values you need. Us these values in your code– Programmer
Jun 30 at 13:00
playerBody.velocity
playerBody.AddForce
Yeh I just watched that video and it wouldnt work in my case because im manipulating its velocity in code. I dont think force would work either because in my example the player moves as a constantly speed and add force would just be like an explosion, shooting the ball. I just didnt want a constant speed if its velocity was below 0 when trying to move right and visa versa for left
– Rachel Dockter
Jun 30 at 13:02
I never said it wouldn't work with velocity. I suggested
AddForce
as an alternative but since that's not ok in your scenario, use velocity. Before you do, I highly suggest you use ConstantForce
. I think that's appropriate in your case than velocity. Now, combine it with Physics Material.– Programmer
Jun 30 at 13:07
AddForce
ConstantForce
oh ok ill try constance force, thanks
– Rachel Dockter
Jun 30 at 13:10
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Thanks ill try this but should i keep the code the same? Wouldnt the code overwrite this? since im explicitly setting the velocity
– Rachel Dockter
Jun 30 at 12:51