How to declare unlimited variables in a loop depending on user input?
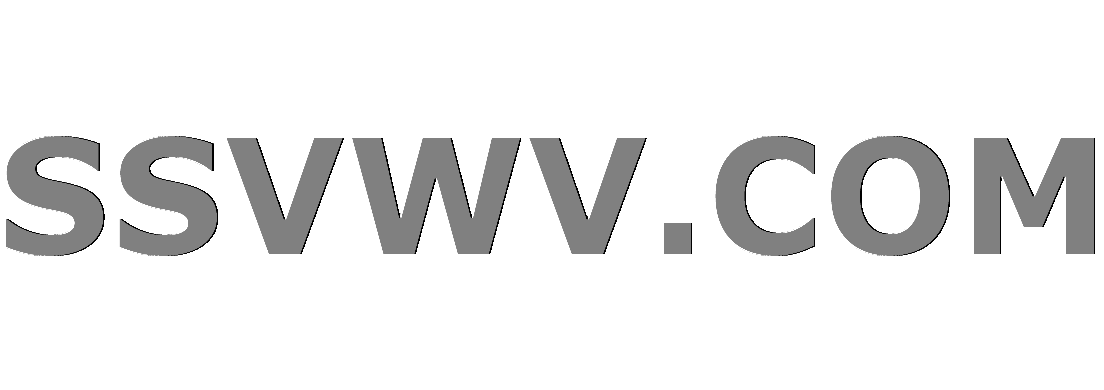
Multi tool use
How to declare unlimited variables in a loop depending on user input?
I am building a program in eclipse and I want to make a loop in which variables are declared depending on user input.
for eg, the first input is stored in a1, next input in a2, next one in a3, and so on until the user types anything other than a number input.hasNextDouble()
.
input.hasNextDouble()
I tried doing this using while
loop, and Scanner
class with if/else
statements. But unable to figure it out. Any help will be appreciated.
while
Scanner
if/else
List
docs.oracle.com/javase/tutorial/collections
– JB Nizet
Jun 30 at 15:00
Good question from an inquisitive mind, but perhaps if you continue through your Java learning material, you'll very soon come to the part where they teach you about Java collections, which includes the
List
as suggested by Jacob.– Andreas
Jun 30 at 15:10
List
Use Array or List.
– Gokul Nath KP
Jun 30 at 15:25
1 Answer
1
You could use a HashMap:
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
class Main {
public static void main(String args) {
Scanner scanner = new Scanner(System.in);
Map<String, Double> inputs = new HashMap<String, Double>();
boolean isNumber = true;
int count = 1;
while(isNumber) {
System.out.printf("Please enter a%d:", count);
if(scanner.hasNextDouble()) {
inputs.put("a" + count, scanner.nextDouble());
count++;
} else {
isNumber = false;
}
}
System.out.println("Inputs: " + inputs);
// Auxiallary code for question in comments below
double sum = 0.0f;
for (double d : inputs.values()) {
sum += d;
}
System.out.println("Sum: " + sum);
for (Map.Entry<String, Double> entry : inputs.entrySet()) {
System.out.println("Sum - " + entry.getKey() + " = " + (sum - entry.getValue()));
}
}
}
Example Usage:
Please enter a1: 5.5
Please enter a2: 6
Please enter a3: 7.2
Please enter a4: x
Inputs: {a1=5.5, a2=6.0, a3=7.2}
Sum: 18.7
Sum - a1 = 13.2
Sum - a2 = 12.7
Sum - a3 = 11.5
Is it possible to interact with the inputs individually? I want to add these inputs, and then subtract each of them from the sum
– Rockboy987
2 days ago
Please see the auxiliary code I have added above to calculate the sum into a variable, and subtract each input from that sum.
– shash678
2 days ago
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You seem to be looking for a
List
.– Jacob G.
Jun 30 at 14:59