In javascript how does the below code works
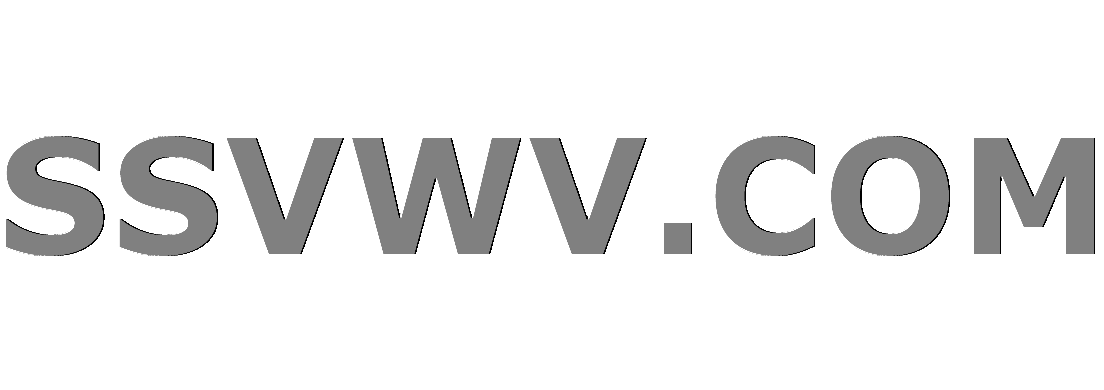
Multi tool use
In javascript how does the below code works
In Javascript how does the below code works.
var a = {
prop1: "a",
prop2: "b",
fun: function() {
return this.prop1 + " " + this.prop2;
}
}
var a2 = a;
a.fn = "v";
a = {};
if (a === a2) {
console.log(true);
} else {
console.log(false);
}
The above code prints false.
But if I comment out the line a={} the value which prints on console is true.
var a = {
prop1: "a",
prop2: "b",
fun: function() {
return this.prop1 + " " + this.prop2;
}
}
var a2 = a;
a.fn = "v";
//a={};
if (a === a2) {
console.log(true);
} else {
console.log(false);
}
How the above code works, as Both variables(a and a2) points to the same object but when I initialized a with {} it gave false.
a
{}
a2
a
{}
var a2=a;
Check this answer to "Is JavaScript a pass-by-reference or pass-by-value language?"
– t.niese
Jun 30 at 17:00
4 Answers
4
...as Both variables(a and a2) points to the same object ...
They don't anymore, as of this line:
a={};
At that point, a2
refers to the old object, and a
refers to a new, different object.
a2
a
a2 = a
doesn't create any kind of ongoing link between the variable a2
and the variable a
.
a2 = a
a2
a
Let's throw some Unicode-art at it:
After this code runs:
var a = {
prop1: "a",
prop2: "b",
fun: function() {
return this.prop1 + " " + this.prop2;
}
}
var a2 = a;
a.fn = "v";
At this point, you have something like this in memory (with various details omitted):
Those "Ref" values are object references. (We never actually see their values, those values are just made up nonsense.) Notice that the value in a
and the value in a2
is the same, however.
a
a2
If you do a === a2
at this point, it will be true
: Both variables refer to the same object.
a === a2
true
But when you do this:
a={};
At this point, a === a2
is false
: The variables refer different objects.
a === a2
false
In first case after this line var a2 = a;
a
is again initialized as an empty object, so now a & a2 are two different reference
var a2 = a;
a
var a = {
prop1: "a",
prop2: "b",
fun: function() {
return this.prop1 + " " + this.prop2;
}
}
var a2 = a;
a = {};
console.log(a, a2)
if (a === a2) {
console.log(true);
} else {
console.log(false);
}
In second case they point to same location, so adding a new property will reflect in the other too.
var a = {
prop1: "a",
prop2: "b",
fun: function() {
return this.prop1 + " " + this.prop2;
}
}
var a2 = a;
a.fn = "v";
console.log(a, a2)
//a={};
if (a === a2) {
console.log(true);
} else {
console.log(false);
}
In the first case :
var a2 = a; // now a2 and a have reference (or point to) the same object"
a= {}; // a now points to new reference
Since both a and a2 points has different references i.e why it returns false in first.
When you comment a= {}
both a and a2 points to same location or stores same reference that is why it returns true.
a= {}
The comment "// now a2 has reference of a or it points to a" is a bit misleading in the exact way the original question was confused. It might be more accurate to say that "now
a2
and a
have reference (or point to) the same object"; a2
does not point at the variable a
, if it did, the a={};
would also change what a2
is pointing to.– Peteris
Jun 30 at 17:34
a2
a
a2
a
a={};
a2
@Peteris thanks, updated :-)
– amrender singh
Jun 30 at 17:36
var a = {
prop1: "a",
prop2: "b",
fun: function() {
return this.prop1 + " " + this.prop2;
}
}
var a2 = a;
a.fn = "v";
a = {};
console.log(a);
console.log(a2);
if (a === a2) {
console.log(true);
} else {
console.log(false);
}
Look at your code carefully. a is not innitialized to {}
. It was initialized in the first line where it says var a =
. I have printed out both values for you. var a2
gets the value of var a, not the reference so when var a changes, var a2 does not change.
{}
var a =
var a2
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
In the first example
a
points to the assigned{}
anda2
to the objecta
hold before{}
was assigned to it.var a2=a;
does not create an alias or reference.– t.niese
Jun 30 at 16:55