Unity serialized objects in different Lists not equal after pressing Play
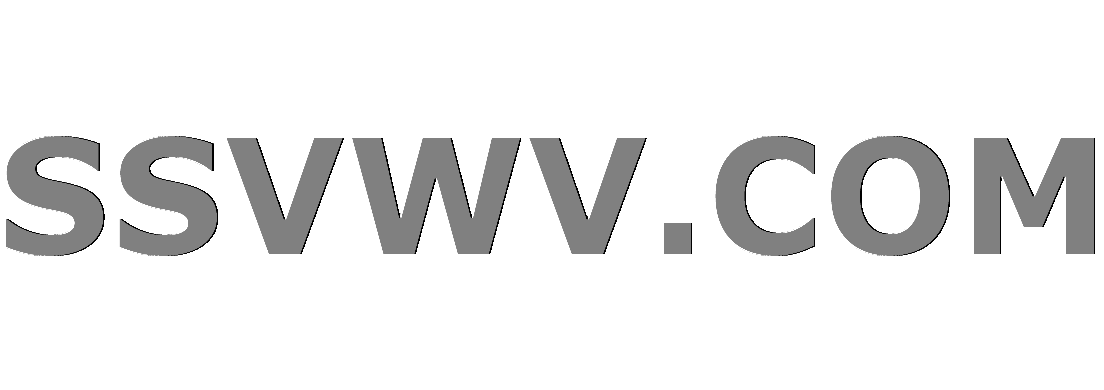
Multi tool use
Unity serialized objects in different Lists not equal after pressing Play
basicly I have these lists:
[System.Serializable]
public class PatrolGraph : MonoBehaviour {
public List<Node> nodes = new List<Node>();
public List<Edge> edges = new List<Edge>();
[...]
}
I generate both Lists (nodes and edges) using a method I run from the editor.
Node and Edge are both serializable, and Edge containes two Nodes. However, after I hit play, the Nodes saved in the Edge objects of the edges list don't seem to be the same as the Nodes in my nodes list (NodeA.Equals(NodeB)), even though they should be.
If I run the function to generate the Lists during Playmode (the same one I run in edit mode), the Equals function suddenly returns true.
Here is the code for the Node and Edge classes:
Node:
[System.Serializable]
public class Node
{
public float lastVisited = 0;
public Vector3 position;
}
Edge:
[System.Serializable]
public class Edge
{
public Node A;
public Node B;
public Edge (Node A, Node B)
{
this.A = A;
this.B = B;
}
}
I want Node A and B in the Edge objects to be the same Nodes as ones saved in the nodes list after I hit play. What am I doing wrong?
Thanks in advance
2 Answers
2
It's because Object.Equals
check only reference when Edge
and Node
are reference types. And Serialialization create new object with new address, that's why Equals
returns false. If you override this method to check values of fields it will solve your problem. I recommend to check MSDN for further information how to override Equals. Or answer on forum with unity community:
Object.Equals
Edge
Node
Equals
@Frager007 you want to keep the same reference value in Edge and list of nodes?
– Kacper
Jun 24 at 21:59
Yes. Node A and B from an Edge need to be references to Nodes in the list nodes. So when I edit Node A in an edge for example, it changes the values in the node list, as well as in other Edge objects
– Frager007
Jun 24 at 22:07
So i think it isn't possible because serialization and deserialization of list of nodes and edges are made apart, so deserialization will create two Nodes with the same value by different reference. To workaround you should write your own serialization or something, or extract Nodes from Edges, or assign Nodes to Edges.
– Kacper
Jun 24 at 22:12
Thanks, If serialisation makes what I want not possible, I will try a workaround and save the nodes only in a list and have the edges save the list indexes of the nodes instead of the nodes themselves.
– Frager007
Jun 25 at 10:53
As mentioned by Kacper, it is not possible to save references when using serialisation. After deserialisation, The Nodes referenced in multiple variables will result in seperate instances for each variable and cause the Equals function to return false when used on those instances.
However I did manage to save the Edges between Nodes by adding ids to the Nodes, that represent their list index
for (int i = 0; i< nodes.Length; i++){
nodes[i].id = i;
}
and having the Edges just save the index/ids of the nodes instead of referencing the nodes themselves
public class Edge
{
public int id_1;
public int id_2;
}
which solved the problem.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Thanks, but overriding Equals dosen't solve my problem (I already tried that). What I want is the Node objects in the Edge objects to be references to the same Node objects i saved in my nodes list. The problem with overriding Equal(I checked for equal position) was that the lastVisited field was different, depending on from where I accesed the Node and that does screw up my patroling algorithm.
– Frager007
Jun 24 at 21:50